file: docs/pages/index.mdx
meta: {
"layout": "landing"
}
import { HomePage } from "vocs/components";
{" "}
The Realmverse is a decentralized, open-source, and community-driven gaming ecosystem built on the Dojo framework,
featuring both Eternum seasonal gameplay and Blitz competitive tournaments.
Get started
Play Now
---
file: docs/pages/blitz/achievements.mdx
meta: {}
# 🏆 Achievements
*Work in Progress...*
---
file: docs/pages/blitz/key-concepts.mdx
meta: {
"title": "Key Concepts",
"description": "The foundational mechanics of Blitz"
}
# 🔑 Key Concepts
Realms: Blitz is a real-time strategy variant of Eternum designed for rapid, competitive play. In each two-hour match,
players strategically manage resources, explore territories, and engage in high-intensity military confrontations.
Blitz's streamlined mechanics and accelerated gameplay require swift decision-making and adaptability, resulting in a
high skill ceiling that rewards skill and experience. The no-stakes Recruit bracket allows new players to learn the
game, while experienced Lords can sharpen their skills or test new strategies in preparation for a Blitz series. Warrior
bracket games, which require a $LORDS entry fee, form the core of each series—where success brings rewards and
qualifications for the coveted Elite games, offering even greater prizes.
## Rule Summary
### Game Duration
Each Blitz game runs for exactly **2 hours**. Eternum Days are 5 minutes in length to support the fast-paced format.
### Realms
* **Three Starting Realms**: Each player starts with three Realms, evenly spaced apart in a triangle formation.
* **Equal Capability**: All Realms in Blitz are functionally identical.
* **Free Building**: Players can construct any resource building they wish, restricted only by build space and
population.
* **No Wonders or Villages**: Wonder bonuses and the Village system are not present in Blitz.
* **Conquest Mechanics**: All Realms are vulnerable to capture by other players, encouraging constant vigilance and
strategic interaction.
### Materials
* **Simplified Resources**: Only 9 resources are available - Wood, Coal, Copper, Ironwood, Cold Iron, Gold, Adamantine,
Mithril, and Dragonhide.
* **Simplified Food**: Only 1 food type - Wheat.
* **Essence**: New material found by exploring the map and interacting with World Structures, required for some
production, buildings and upgrades.
* **Labor**: Required for all construction and 'simple mode' production, acquired through burning resources.
* **Donkeys**: Required for moving materials around the world, essential for logistics.
* **Troops**: 3 types (Knights, Crossbowmen, Paladins), 3 tiers (T1, T2, T3).
* **Restricted Bridging**: Players may only bridge Relics into the game (not out).
* **Donkey Cost**: Donkeys only cost food to produce (no $LORDS cost).
* **No P2P Transfers**: No direct transfers or trade between players.
### Buildings
* **Construction**: Standard and simple mode building systems.
* **Building Types**:
* **The Keep**: Central structure automatically constructed at game start.
* **Resource Buildings**: Specific buildings for each of the 9 resources.
* **Economic Buildings**: Includes Farms, Worker Huts, Storehouses, and Markets.
* **Military Buildings**: Nine different buildings for producing three tiers of each troop type.
* **Placement & Population**: Buildings require buildable hexes and population capacity, with more unlocked as Realms
are upgraded.
### Production
* **Production Types**:
* **Food**: Wheat can be produced without inputs.
* **Resources**: Require combinations of other resources (standard) or labor (simple).
* **Labor**: Produced by burning resources in the Keep.
* **Troops**: Essential for exploration and warfare, with production of higher tier troops requiring lower tier troops
as inputs.
* **Donkeys**: One-time-use transport, consumed after a single journey.
* **Altered Rates**: All production rates are increased relative to production requirements for the fast-paced game
format.
### World Map
* **Exploration System**: Players must explore unrevealed hexes to find Essence, claim Hyperstructures, and attack
neighbors.
* **No Resource Discovery**: Players will no longer find resources when exploring.
* **Essence Discovery**: Players primarily find Essence when exploring, along with small stacks of donkeys, T1 troops
and labor.
* **Relic Discovery**: Powerful Relics can be found in Relic Crates that occasionally appear on the world map.
* **Biomes**: 16 unique biome types affecting combat effectiveness and troop movement.
* **Movement & Stamina**: Armies move using stamina (regenerating at +60 per Eternum Day), with costs varying by biome
and troop type.
### Military
* **Army Composition**: Armies consist of a single troop type and tier.
* **Troop Tiers**: T1, T2, and T3 troops with increasing stamina and combat strength.
* **Combat Factors**: Battles are resolved based on army damage, stamina modifiers, and biome effects.
* **Army Types**:
* **Field Armies**: Deploy onto the world map for exploration and conquest.
* **Guard Armies**: Deployed to defense slots to protect structures.
* **Stamina System**: Armies recover +60 stamina each Eternum Day.
* **Biome Effects**: Each troop type has advantages (+30% damage), disadvantages (-30% damage), or no change (0%) in
specific biomes.
* **Reinforcement Movement**: Players can't transport troops by donkey to World Structures - reinforcements must be
moved across the map from a Realm.
### Scoring System
Leaderboard placing is determined by total accumulated Victory Points, a measure of a Lord's ability to explore the map
and control World Structures. Victory Points are awarded for:
* Exploring tiles
* Claiming World Structures
* Claiming Hyperstructures
* Holding Hyperstructures throughout the game
### Victory
Blitz uses a scoring-based system rather than victory points. The players with the highest Total Score on the
leaderboard at the end of the two hours share in the $LORDS prize pool. This pool is made up of the cumulative entry
fees that players pay to enter the game.
## Blitz Brackets
### Recruit Bracket
* **Low Stakes**: Free-to-enter bracket for introduction and practice.
* **No Entry Cost**: No $LORDS required to participate.
* **Prizes**: Relic Chests only.
* **Frequency**: Games run every day on varying schedules to encourage global participation.
### Warrior Bracket
* **Medium Stakes**: Competitive games requiring a $LORDS entry fee.
* **$LORDS Entry Cost**: $LORDS fee contributes to shared prize pool.
* **Prize Distribution**: Top 15% of players share the prize pool.
* **Rewards**: Relic Chests and Blitz Rewards Chests.
* **Special Reward**: Highest placing players win tradable NFT invites to Elite bracket.
* **Frequency**: Games run a few times weekly during prime time for key timezones (USA, Europe, Asia-Pacific).
### Elite Bracket
* **High Stakes**: Highest level of Blitz competition.
* **Ticket Entry Cost**: NFT invite from Warrior bracket (no additional $LORDS cost).
* **Prize Pool**: Large, DAO-funded prize pool (pending BIP).
* **Rewards**: Relic Chests, Blitz Rewards Chests and Elite Blitz Rewards Chests.
* **Frequency**: Games run at the end of a Blitz series.
### Blitz Series
While Recruit games can be deployed and played as frequently as the player base demands, games with stakes
(Warrior/Elite) are organized into 'series'. Each series takes place between Eternum seasons to bridge the gap in
gameplay and provide continuous competitive opportunities.
## Rewards
Successful Blitz players can earn various rewards including:
* **$LORDS Token**: Prize pool distributions for top players.
* **Loot Chests**: Relic Chests, Blitz Rewards Chests and Elite Blitz Rewards Chests containing consumables and cosmetic
items.
* **Entry Tickets**: NFT invites to Elite bracket games for top Warrior bracket performers.
---
file: docs/pages/blitz/prize-pool.mdx
meta: {}
# 💰 Prize Pool
*Work in Progress...*
---
file: docs/pages/blitz/victory.mdx
meta: {}
import { table } from "@/components/styles";
# 👑 Victory
## Scoring System
Leaderboard placing is determined by total accumulated Victory Points (VP), a measure of a Lord's ability to explore the
map and control World Structures. Victory Points are awarded for:
* Exploring tiles
* Claiming World Structures (Essence Rifts and Camps)
* Claiming Hyperstructures
* Holding Hyperstructures throughout the game
*Note: Wolrd Structure claim VP are only rewarded the first time a World Structure is captured (from bandit forces).*
The number of VP awarded for each feat described above is as follows:
Action |
VP Awarded |
Explore a tile |
5 VP |
Claim a World Structure from bandits |
200 VP |
Claim a Hyperstructure from bandits |
500 VP |
Control a Hyperstructure |
1 VP/second |
---
file: docs/pages/blitz/world-physics.mdx
meta: {}
import { Biomes } from "@/components/Biomes";
# ⚛️ World Physics
In Blitz, every action is governed by onchain physics—immutable rules encoded directly onto the blockchain, ensuring
fairness, transparency, and absolute consistency for all Lords. Here, code is law; no exceptions exist outside the
boundaries set by these protocols.
## Game Duration
Each Blitz game runs for exactly **2 hours**.
## Time
Time within a Blitz game is measured in Eternum Days, and each is precisely 5 minutes in length. One day ends and the
next begins every 5 minutes, so a single Blitz game takes place over 24 Eternum Days.
## Materials
In Blitz, materials such as food, resources, troops and donkeys are isolated within each round. There is no bridging of
materials in and out of the game (other than Relics, which can be bridged in prior to the start of the game). All
materials have an associated weight, which determines the amount that can be stored and the number of donkeys required
to move the goods from place to place.
*Note: This document will use 'materials' to refer to all tangible assets - i.e. food, resources, essence, labor,
troops, and donkeys. This is not to be confused with 'resources', which specifically refers to the 9 resources in
Blitz.*
## Cost of Production
With the exception of food, nothing in Blitz is produced for free; every unit of production demands an input—food,
labor, resources, or some combination the three. Lords must consider these costs and effectively balance production to
ensure they can maximize their Score within the 2-hour game window.
## Travel
Transporting materials takes time and donkeys. Every transfer of materials between locations relies exclusively on these
speedy and dependable creatures. Donkeys are single-use, consumed upon completing their journey, making continuous
acquisition vital for maintaining supply chains.
Troops can also be moved between Realms using donkeys, but to dominate your enemies and ensure security of your lands,
troops must be deployed to an army on the world map. Once troops are deployed, they cannot be taken back into the
inventory. Their mobility is then governed by stamina, limiting the distance they can travel and the number of actions
they can perform. Traveling between hexes or initiating an attack expends valuable stamina points, which are partially
replensished each Eternum Day.
## Hexes
Blitz unfolds across a procedurally-generated, hexagonal-tile world map. This represents the physical space of the world
and defines spawn locations, biome distribution, and distances between Realms and other World Structures. This is the
layer in which armies explore, fight, and capture, and is explorable in the 'World' view.
Zooming in, each Realm hex is broken up into smaller hexes which define the local area and building placement. Here in
the 'Local' view, Lords can plan out their building placement and closely manage their population, supply chain, and
production.
## Biomes
Each hexagonal tile on the world map possesses a distinct biome. Each troop type responds uniquely to these varied
terrains, gaining or losing combat efficiency and stamina based on their affinity with different biomes. Successful
Lords must master biome knowledge to exploit strategic advantages or avoid costly missteps.
## The Blitz Arena
The map on which Blitz takes place is designed to position Lords as equally as possible while also providing a dynamic
battlefield that scales appropriately. Each player starts the game with three Realms, evenly spaced apart in a triangle
and positioned between three complete and revealed Hyperstructures. Player Realms and Hyperstructures are placed in a
specific pattern to ensure that all Lords have a comparable number of neighbors and equal access to Hyperstructures.
More detail on the Realm and Hyperstructure placement systems are available in the [Realms](/blitz/realms/realm) and
[World Map](/blitz/worldmap-movement/worldmap) sections.
The primary objective of Blitz is to capture and hold as many Hyperstructures and World Structures as possible to
accumulate Victory Points and secure a position at the top of the leaderboard.
---
file: docs/pages/blitz/world-structures.mdx
meta: {}
# 🏗️ World Structures
All World Structures in Blitz are initially occupied by bandit forces that must be defeated by a player before it can be
claimed.
## Hyperstructures
In Blitz, Hyperstructures are **fully constructed** and positioned around the map at the start of the game, ready to be
claimed by eliminating resident bandit forces. There is no construction process - Hyperstructures are immediately
functional once claimed and will begin accumulating Score for the owning player.
The number of Hyperstructures in the game, and their positioning on the world map, is explained in the
[World Map](/blitz/worldmap-movement/worldmap) section.
## Essence Rifts
Essence Rifts are striking fissures in the earth where valuable Essence has swelled from beneath the surface. These
locations provide a steady source of the valuable dust to the owning player, reducing the need to explore new hexes for
continued Realm expansion and troop production. Essence Rifts produce Essence at a rate of 1 unit per second.
## Camps
Camps act in a similar capacity to Villages in Eternum, in the sense that they perform as small, discoverable Realms
that can boost production and act as forward bases. Camps are restricted to the Settlement level and cannot be upgraded.
---
file: docs/pages/development/client.mdx
meta: {}
# React Client
## Run the dev server
From the root of the project, run:
Easy method:
```bash
./scripts/client.sh
```
Manual method:
```bash
pnpm i && pnpm dev
```
The client requires environment variables to be set. See the [Environment Variables](client/.env.sample) page for more
information.
## Project Structure
### Elements
* Small reusable react UI components
### Components
* Stateful react components composed of Elements. This is where all the onchain state should be stored.
### Modules
* Collection of components composed into Modules
### Containers
* Locations where Modules are composed into a full page
### Layouts
* Collection of containers composed into a full layout
## useDojo Hook
The `useDojo` hook is a core part of the Dojo framework that provides access to essential game functionality, account
management, and network interactions.
### Usage
```tsx
import { useDojo } from "@/hooks/context/dojo-context";
function GameComponent() {
const { account, network, masterAccount } = useDojo();
// ... your game logic
}
```
### Features
#### Account Management
The hook provides comprehensive wallet and account management capabilities:
* **Burner Wallet Creation**: Create temporary wallets for players
* **Account Selection**: Switch between different burner wallets
* **Account Listing**: View all available burner wallets
* **Master Account Access**: Access to the game's master account
* **Deployment Status**: Track wallet deployment status
#### Network Integration
Access to network configuration and setup results for interacting with the Starknet blockchain.
#### Game Setup
Provides access to all initialized game systems, components, and world configurations.
### Requirements
The hook must be used within a `DojoProvider` component and requires the following environment variables:
* `VITE_PUBLIC_MASTER_ADDRESS`
* `VITE_PUBLIC_MASTER_PRIVATE_KEY`
* `VITE_PUBLIC_ACCOUNT_CLASS_HASH`
### Return Value
The hook returns an object containing:
* `setup`: Complete Dojo context including game systems and components
* `account`: Account management functions and state
* `network`: Network configuration and setup results
* `masterAccount`: The master account instance
### Example
```tsx
function GameUI() {
const { account } = useDojo();
return (
Current Account: {account.account.address}
);
}
```
## Eternum provider
Wrapper around the DojoProvider, which itself is a wrapper around a generic Starknet provider, an API to easy interact
with your contract’s API via the RPC. Each layer of abstraction adds its own set of functionalities, with the Eternum
Provider mainly providing easy access to all the system calls that our systems expose (create\_army, etc…)
## Offchain messages
You can use offchain messages to store information in the indexer, but it is not persisted onchain. Some examples of use
cases for this are in-game messages. Refer to
[this code](https://github.com/BibliothecaDAO/eternum/blob/1490dfcf513d72f51e5a84138be743d774338caf/client/src/ui/modules/chat/InputField.tsx#L50)
for an example of how to use offchain messages
# State Management with Recs
Recs allow you to query the state of the world and subscribe to changes in the world. It is the recommended way to
manage state in the client.
```tsx
const structureAtPosition = runQuery([HasValue(Position, { x, y }), Has(Structure)]);
```
This line of code will run a query against the local state of the browser and return all the structures that are at the
position `{ x, y }`. This does not execute any call to Torii as the local state is already synced with the latest
updates.
This part of the code in `client/src/dojo/setup.ts` is where the local state is initialized and synced.
## Subscribing to changes
To subscribe to changes in the world, you can use the `useEntityQuery` hook. This hook will return the entity that
matches the query and update it if it changes.
```tsx
const allRealms = useEntityQuery([Has(Realm)]);
```
## Getting the value of your components
After you have run a query, you can get the value of your components using the `getComponentValue` function.
```tsx
const realm = getComponentValue(Realm, entityId);
```
The entityId is the poseidon hash of all the keys of the component you want to get. This can be an easier way to get
your component than using a query if you already know all the keys.
### Getting a component from an entity
If you have an entityId, you can get a component from it using the `getComponent` function.
```tsx
const realm = getComponentValue(Realm, getEntityIdFromKeys([entityId]));
```
The previous line of code is equivalent to:
```tsx
const entity = runQuery([Has(Realm), HasValue(Keys, [entityId])]);
const realm = getComponentValue(Realm, Array.from(entity)[0]);
```
# Extending the client
## Adding a new component
You will need to add your component to the `contractComponents.ts` file. This will ensure that the component is synced
with the state of the world and will provide the types for the component.
## Adding a new system
You will need to add your system logic to the `sdk/packages/eternum/src/provider/index.ts` file and then use it in the
`createSystemCalls.ts` file. This will ensure that the system is called with the correct arguments and will provide the
types for the system.
## Optimistic updates
You can use optimistic updates to update the local state without waiting for the transaction to be included in a block.
This is useful to provide a better user experience by updating the UI immediately.
```ts
private _optimisticDestroy = (entityId: ID, col: number, row: number) => {
const overrideId = uuid();
const realmPosition = getComponentValue(this.setup.components.Position, getEntityIdFromKeys([BigInt(entityId)]));
const { x: outercol, y: outerrow } = realmPosition || { x: 0, y: 0 };
const entity = getEntityIdFromKeys([outercol, outerrow, col, row].map((v) => BigInt(v)));
this.setup.components.Building.addOverride(overrideId, {
entity,
value: {
outer_col: outercol,
outer_row: outerrow,
inner_col: col,
inner_row: row,
category: "None",
produced_resource_type: 0,
bonus_percent: 0,
entity_id: 0,
outer_entity_id: 0,
},
});
return overrideId;
};
```
You can use the `uuid` function to generate a unique overrideId. Remember to return the overrideId so you can later
delete it after the transaction is included in a block. It's good practice to remove the override, however because of a
delay between the transaction being included in a block and Torii syncing, you might have a split second where the
override is removed and the Recs being updated. This will cause your component (i.e. a building) to appear then
disappear for a second before reappearing.
---
file: docs/pages/development/collaborators.mdx
meta: {}
import Collaborators from "@/components/Collaborators";
# Collaborators
---
file: docs/pages/development/contracts.mdx
meta: {}
# Contracts
## Rules of thumb
* Note that every field you add in a model will raise the storage cost of each transaction that modifies the model.
* Try to make models as small as possible, and re-use existing models as much as possible, every model and system that
you add raises the size of the CASM, and there is a limit to the size of a declared contract on Starknet.
* Keep systems stateless. Store your game state in models.
* When implementing a new game logic, you will need to keep in mind that the game already has physics implemented, such
as weight, position, movement, speed, etc. If creating something that needs to have any of this logic, re-use
components so everything stays logical.
## Models
### Keys
Use the `ID` type alias for keys that require a unique identifier.
### Model design
entity\_id: u32
### Implementations
Where possible make traits for the models so they are stateless for unit testing.
Always use generate trait where possible to minimise code.
```rust
#[generate_trait]
```
### Adding a model
```rust
#[derive(Copy, Drop, Serde)]
#[dojo::model]
pub struct Structure {
#[key]
entity_id: ID,
category: StructureCategory,
created_at: u64
}
```
To test this model, you need to add it to the `contracts/src/utils/testing/world.cairo` file so it can be instantiated
in the tests.
## Event models
If you need some data to be available in the client, but it doesn't need to be stored onchain, you can use an event
model.
```rust
#[derive(Introspect, Copy, Drop, Serde)]
#[dojo::event]
#[dojo::model]
pub struct ExampleEvent {
#[key]
id: ID,
#[key]
event_id: EventType,
my_data_field: u8
}
```
## Adding a system
Design systems like this in the directory
* SystemName
* system\_name.cairo
* tests.cairo
`system.cairo` should include the implementation of the system like this.
Things to note:
* Interface at top of File
* use of `super::IBuildingContract` to minimise imports and make it clear where the interface is defined.
```rust
#[dojo::interface]
trait IBuildingContract {
fn create(
entity_id: ID,
building_coord: s1_eternum::models::position::Coord,
building_category: s1_eternum::models::resource::production::building::BuildingCategory,
produce_resource_type: Option
);
}
#[dojo::contract]
mod production_systems {
use s1_eternum::alias::ID;
use s1_eternum::models::{
resource::resource::{Resource, ResourceCost}, owner::Owner, hyperstructure::HyperStructure,
order::Orders, position::{Coord, Position, PositionTrait, Direction},
buildings::{BuildingCategory, Building, BuildingImpl},
production::{Production, ProductionRateTrait}, realm::{Realm, RealmImpl}
};
#[abi(embed_v0)]
impl BuildingContractImpl of super::IBuildingContract {
fn create(
world: IWorldDispatcher,
entity_id: ID,
building_coord: Coord,
building_category: BuildingCategory,
produce_resource_type: Option,
) {
}
}
}
```
To test this system, you need to add it to the `contracts/src/utils/testing/world.cairo` file so it can be instantiated
in the tests.
---
file: docs/pages/development/getting-started.mdx
meta: {
"layout": "docs"
}
# Getting Started
## Prerequisites
* [Dojo onchain game engine](https://book.dojoengine.org) v1.0.4
* [Node.js](https://nodejs.org/)
* [pnpm](https://pnpm.io/) v9.12.3
* [Bun](https://bun.sh/)
## Setup
Install Dojo via:
```bash
curl -L https://install.dojoengine.org | bash
```
Eternum uses a pnpm workspace and bun for scripts to allow easy npm packages to be created. Install pnpm:
```bash
npm install -g pnpm
```
Install project dependencies:
```bash
pnpm install
```
Build shared packages:
```bash
pnpm run build:packages
```
## Development Scripts
### Development
* `pnpm dev` - Start game development server
* `pnpm dev:docs` - Start documentation development server
* `pnpm dev:landing` - Start landing page development server
### Building
* `pnpm build` - Build game client
* `pnpm build:docs` - Build documentation
* `pnpm build:landing` - Build landing page
* `pnpm build:packages` - Build shared packages
### Testing & Linting
* `pnpm test` - Run all tests
* `pnpm lint` - Run linting
* `pnpm format` - Format code
* `pnpm format:check` - Check code formatting
## Contract Deployment
Eternum supports multiple deployment environments:
| Environment | Description |
| ----------- | --------------------------- |
| Local | For development and testing |
| Slot | Staging environment |
| Sepolia | Public testnet |
| Mainnet | Production environment |
### Deploying to Local
Before deploying to any environment, confirm that you have a `.env.{environment}` file in the `contracts/common`
directory, as well as in the `client/apps/game` directory.
To deploy and run the game locally:
```bash
# Start local game contracts
pnpm run contract:start:local
```
### Deploying to Sepolia
To deploy the contracts to Sepolia testnet, run these commands in order:
1. Deploy game contracts:
```bash
pnpm run game:migrate:sepolia
```
2. Deploy season pass contracts:
```bash
pnpm run seasonpass:deploy:sepolia
```
3. Deploy season resources contracts:
```bash
pnpm run seasonresources:deploy:sepolia
```
4. Update TOML configuration:
```bash
pnpm run toml:update:sepolia
```
5. Start the indexer:
```bash
pnpm run indexer:start:sepolia
```
6. Deploy game configuration:
```bash
pnpm run config:deploy:sepolia
```
## Environment Variables
For local development, create a file called `.env.local` in the client repository and add the following:
```bash
VITE_PUBLIC_MASTER_ADDRESS="0x127fd5f1fe78a71f8bcd1fec63e3fe2f0486b6ecd5c86a0466c3a21fa5cfcec"
VITE_PUBLIC_MASTER_PRIVATE_KEY="0xc5b2fcab997346f3ea1c00b002ecf6f382c5f9c9659a3894eb783c5320f912"
VITE_PUBLIC_ACCOUNT_CLASS_HASH="0x07dc7899aa655b0aae51eadff6d801a58e97dd99cf4666ee59e704249e51adf2"
VITE_PUBLIC_FEE_TOKEN_ADDRESS=0x49d36570d4e46f48e99674bd3fcc84644ddd6b96f7c741b1562b82f9e004dc7
VITE_PUBLIC_TORII="http://127.0.0.1:8080"
VITE_PUBLIC_NODE_URL="http://127.0.0.1:5050"
VITE_PUBLIC_GAME_VERSION="v1.0.0-rc0"
VITE_PUBLIC_GRAPHICS_DEV=false
VITE_PUBLIC_TORII_RELAY="/ip4/127.0.0.1/udp/9091/webrtc-direct/certhash/uEiDry6d-bDv3UdIS6L9VMugoiZnfwqOeXyFWm6jgKf7aTw"
VITE_SOCIAL_LINK=http://bit.ly/3Zz1mpp
VITE_PUBLIC_CHAIN=local
VITE_PUBLIC_SLOT="eternum-prod"
VITE_PUBLIC_VRF_PROVIDER_ADDRESS="0x0"
VITE_PUBLIC_CLIENT_FEE_RECIPIENT=0x045c587318c9ebcf2fbe21febf288ee2e3597a21cd48676005a5770a50d433c5
VITE_PUBLIC_MOBILE_VERSION_URL=https://next-m.eternum.realms.world
```
This file is subject to change, please keep an eye on the repository for potential updates.
## Project Structure
* [Client](https://github.com/BibliothecaDAO/eternum/tree/main/client) - React apps built with Vite
* [Game](https://github.com/BibliothecaDAO/eternum/tree/main/client/apps/game) - Main game client with Three.js
interface
* [Landing](https://github.com/BibliothecaDAO/eternum/tree/main/client/apps/landing) - Landing page
* [Game Docs](https://github.com/BibliothecaDAO/eternum/tree/main/client/apps/game-docs) - Game documentation
* [Balancing](https://github.com/BibliothecaDAO/eternum/tree/main/client/apps/balancing) - Game balancing tools
* [Contracts](https://github.com/BibliothecaDAO/eternum/tree/main/contracts) - Cairo/Dojo smart contracts
* Game contracts
* Season Pass contracts
* Season Resources contracts
* [Packages](https://github.com/BibliothecaDAO/eternum/tree/main/packages) - Shared libraries
* [Core](https://github.com/BibliothecaDAO/eternum/tree/main/packages/core) - Eternum SDK
* [React](https://github.com/BibliothecaDAO/eternum/tree/main/packages/react) - React hooks and components
* [Config](https://github.com/BibliothecaDAO/eternum/tree/main/config) - Configuration and deployment scripts
## Core Dependencies
* [@dojoengine/react](https://www.npmjs.com/package/@dojoengine/react) - React integration for Dojo
* [@dojoengine/recs](https://www.npmjs.com/package/@dojoengine/recs) - Entity Component System
* [@cartridge/controller](https://www.npmjs.com/package/@cartridge/controller) - Game controller integration
* [Starknet.js](https://www.npmjs.com/package/starknet) v6.23.1 - StarkNet interaction
* [Vite](https://vitejs.dev/) - Frontend build tool
## Stack
* [Contracts](/development/contracts): built using the Dojo framework. This leverages the ECS model, allowing us to
build quick and ship fast. It revolves around 3 units: Entities, Components (called Models in Dojo) and Systems (more
about this in the Dojo book).
* [Client](/development/client): our client is built using React with Typescript. We leverage Dojo by using DojoJS
* [Eternum SDK](/development/sdk): our SDK contains most notably the getter and setter of the configuration of Eternum,
the EternumProvider and most of the constants/types of the world that needed to be transcribed to Typescript.
* [Torii](https://book.dojoengine.org/toolchain/torii): Dojo's indexer, optimized for that particular framework and well
integrated into the stack. From our client, we have a gRPC subscription set up through DojoJS and recs to events.
Events are fired in the contracts as a way for the indexer to know what's going on in the world. Every time an entity
is created, updated, or deleted, an event is fired which updated Torii's record of the world.
* [DojoJS and recs](https://github.com/dojoengine/dojo.js?tab=readme-ov-file#contributing-to-dojojs): DojoJS is the SDK
that allows easy integration of a Dojo app into your client/node backend. Recs are DojoJS's way of exposing the state
of the world to the client. This is done via queries to the Recs state using a simple query language, which doesn't
support complex queries yet (e.g. joins, gt, lt, etc...).
---
file: docs/pages/development/index.mdx
meta: {
"layout": "docs"
}
# Development
* [Getting Started](/development/getting-started)
* [Client](/development/client)
* [Contracts](/development/contracts)
* [SDK](/development/sdk)
* [Collaborators](/development/collaborators)
---
file: docs/pages/development/llm.mdx
meta: {
"title": "LLM"
}
# LLM
These docs are experted at [https://docs.eternum.realms.world/llm.txt](https://docs.eternum.realms.world/llm.txt) for
consumption by the LLM.
---
file: docs/pages/development/sdk.mdx
meta: {}
# SDK
## Compiling
From the root of the project, run:
```bash
pnpm i && pnpm run build:packages
```
## Structure
This SDK contains most notably the getter and setter of the configuration of Eternum, the EternumProvider and most of
the constants/types of the world that needed to be transcribed to Typescript.
Everything considered as client agnostic should be in there. The main reason for this separation is to be able to re-use
this logic in any other project that uses Typescript, e.g. new mobile client, data analytics app, etc.
---
file: docs/pages/eternum/achievements.mdx
meta: {}
# Achievements & Quests
*WIP*
---
file: docs/pages/eternum/key-concepts.mdx
meta: {
"title": "Key Concepts",
"description": "The foundational mechanics of Eternum"
}
# 🔑 Key Concepts
This section outlines the core mechanics and systems that form the foundation of Eternum's gameplay.
## Realms
* **Limited Assets**: 8,000 unique and irreplaceable Realms serve as the foundation of Eternum, each has the ability to
freely mint a Season Pass.
* **Settling**: Players must burn a Season Pass to settle their Realm in the game world.
* **Starting Materials**: Each newly settled Realm begins with a set of starting materials.
* **Progression Path**: Realms can upgrade from Settlements → Cities → Kingdoms → Empires, unlocking additional
buildable hexes and defensive army slots.
* **Resource Production**: Each Realm can produce 1-7 different resources based on the original Realms NFT metadata.
* **Wonders**: Realms with Wonders have a 20% production bonus and share this bonus with all Realms and Villages in a 12
tile radius.
* **Hardcore Gameplay**: If a Realm’s defenses crumble, it can be claimed by another player—transferring gameplay rights
to the conqueror.
## Villages
* **Accessible Entry**: Villages can be established around settled Realms, offering a simple entry system for new
players
* **Scaling**: Up to 6 Villages per Realm, allowing for 48,000 Villages in Season 1
* **Limited Production**: Produce materials at 50% the rate of Realms and only have access to 1 resource type each
* **Parent Realm**: Each Village is tied to a parent Realm and will pay bridging fees if moving materials out of the
game
* **Troop Restrictions**: Villages may only receive tokenized troops from their parent Realm, they cannot buy or be sent
troops from any other source
* **Simple Progression**: Villages have a limited upgrade path from Settlement to City
* **Casual Gameplay**: Villages can be raided, but can’t be claimed by other players.
## Materials
* **Materials Categories**:
* **Food**: (Wheat, Fish) - essential for production, troop movement, and construction.
* **Resources**: 22 different resource types, distributed based on rarity across both Realms and Villages.
* **Troops**: Units with unique properties that can be assigned to armies.
* **Donkeys**: Required for moving materials around the world, essential for logistics.
* **Labor**: A new material in S1, allows players to build and produce in 'simple mode', acquired through burning
resources.
* **Ancient Fragments**: Found in game, critical for the construction of Hyperstructures.
* **ERC20 Compatibility**: All materials (except Labor) can be traded freely and bridged out as ERC20 tokens through
Realms.
## Buildings
* **Construction Modes**: Two building systems available - Standard (resource-efficient) and Simple (labor-focused).
* **Building Types**:
* **The Keep**: Central structure automatically constructed when settling, provides baseline population capacity.
* **Resource Buildings**: Specific buildings for each of the 22 resources, only requiring food to construct.
* **Economic Buildings**: Includes Farms, Fishing Villages, Worker Huts, Storehouses, and Markets.
* **Military Buildings**: Nine different buildings for producing three tiers of each troop type.
* **Placement & Population**: Buildings require buildable hexes and population capacity, with more unlocked as Realms
and Villages are upgraded.
## Production
* **Production Modes**: Like buildings, production can be done in Standard (resource-intensive) or Simple (food and
labor) mode.
* **Production Types**:
* **Food**: The only resources (Wheat, Fish) that can be produced without inputs.
* **Resources**: Require combinations of other resources (standard) or labor (simple).
* **Labor**: Produced by burning resources in the Keep, used for simple mode construction and production.
* **Troops**: Essential for exploration and warfare, with higher tiers requiring lower tier troops as inputs.
* **Donkeys**: One-time-use transport, consumed after a single journey.
## World Map
* **Exploration System**: The world begins shrouded in mystery, with only the six Banks visible at season start;
adjacent hexes are revealed when settling, and armies can explore to reveal new areas.
* **Biomes**: 16 unique biome types procedurally generated across the map, each affecting combat effectiveness and troop
movement.
* **Movement & Stamina**: Armies move using stamina (regenerating at +20 per Eternum Day), with costs varying by biome
and troop type; Knights, Crossbowmen, and Paladins each have different maximum stamina capacities.
* **Exploration Mechanics**: Exploring costs 30 stamina per hex and reveals that area permanently to all Lords;
exploring armies may discover resources or encounter world structures and agent armies.
## Military
* **Army Composition**: Armies consist of a single troop type and tier (Knights, Crossbowmen, or Paladins).
* **Troop Tiers**: T1, T2, and T3 troops with increasing combat strength.
* **Combat Factors**: Battles resolved based on army damage, stamina modifiers, and biome effects.
* **Army Types**:
* **Field Armies**: Deploy onto the world map for exploration and conquest.
* **Guard Armies**: Deployed to defense slots to protect structures.
* **Stamina System**: Armies recover +20 stamina daily, with 30+ stamina needed for full combat effectiveness.
* **Biome Effects**: Each troop type has advantages (+30% damage), disadvantages (-30% damage), or no change (0%) in
specific biomes.
* **Raiding Mechanics**: Armies can attempt to steal resources from structures without defeating defenders.
* Success depends on the raiding army's damage relative to the combined guard armies.
* Undefended structures can be raided without casualties.
## Tribes
Tribes are player-formed organizations enabling cooperation and coordination. They can be made public (open to all) or
private (invitation only) and allow players access to tribe-only features. Tribes are an early form of social structures
within Eternum and rely on player-driven interactions including arrangements of alliances and declarations of war.
## Victory Conditions
Eternum's seasonal gameplay culminates in victory through the accumulation of victory points earned through the
construction and ownership of Hyperstructures. However, there are various achievement paths, with players earning
recognition and rewards for various actions within the Season.
---
file: docs/pages/eternum/prize-pool.mdx
meta: {}
import { table } from "@/components/styles";
# 💰 Prize Pool
## Overview
In Season 1 of Eternum, there are four prize categories with a total of:
* 1,000,000 $LORDS tokens
* 100,000 $STRK tokens
> **Note:** All Season 1 prizes will be distributed manually by the development team (except for the agent rewards which
> are collected directly in-game). Future seasons will implement automated distribution through game contracts, aligning
> with decentralized gaming principles.
## Prize Categories
### 1. Victory Prizes
**Total: 300,000 $LORDS + 50,000 $STRK**
These prizes are awarded to top-performing tribes based on victory points. Additionally, 2.5% of all $LORDS bridging
volume is collected as a fee and added to the prize pool, further increasing the total rewards available.
#### How to Earn Victory Points
Players can earn victory points by:
* Contributing resources and labor to hyperstructure construction
* Holding shares in completed hyperstructures to accumulate emitted victory points
#### Distribution
Once a player triggers the end of the game (or the game contract breaks), a snapshot of tribes, their members, and the
victory point leaderboard is taken. Tribes will then be ranked by the cumulative number of victory points of their
players. The top 10 tribes receive prizes based on this allocation:
Rank |
Allocation |
1st Place |
30% |
2nd Place |
18% |
3rd Place |
12% |
4th Place |
9% |
5th Place |
7% |
6th Place |
6% |
7th & 8th Place |
5% each |
9th & 10th Place |
4% each |
#### Individual Distribution
Within each tribe:
* Tribe Leader: 30% of tribal allocation (regardless of their victory points)
* Other Members: 70% split proportionally based on victory points contribution to the tribal total
### 2. Achievement Prizes
**Total: 300,000 $LORDS**
Players earn achievement points by completing quests—tracked inside your Cartridge profile, and accessible via your
Cartridge wallet in-game. At the end of the season, this prize pool will be split proportionally amongst all players
based on the number of achievement points they have accumulated.
> **Note:** Achievement details, requirements, and point allocations will be withheld until the game begins to prevent
> planned farming and extractive behaviors. Check the Achievements section for updates when this information becomes
> available.
### 3. Daydreams Agent Prizes
**Total: 250,000 $LORDS + 25,000 $STRK**
#### Agent Rewards
Each agent on the world map carries 10-35 $LORDS, obtainable through:
* Collaboration (persuasion via chat)
* Combat victory
#### Special Achievement
25,000 $STRK will be distributed to the first 100 players who complete the 'Nexus-6' achievement by defeating 10 agent
armies.
### 4. Eternum Arts & Emissaries Prizes
**Total: 150,000 $LORDS + 25,000 $STRK**
Awarded to players or groups making exceptional cultural, artistic, or creative contributions to Eternum. These awards
can be given during or after the game at the developers' discretion.
> **Note:** For more information, join our [Discord community](https://discord.gg/KJff5Ecp).
## Unused Prize Distribution
If any prizes remain unallocated at the end of the game and prize distribution phase:
* Unused $LORDS will be distributed through the veLORDS protocol
* Unused $STRK will be retained by developers for future use
---
file: docs/pages/eternum/tribes.mdx
meta: {}
# 👥 Tribes
Tribes are player-formed organizations enabling cooperation and coordination. They can be made public (open to all) or
private (invitation only) and allow players access to tribe-only features. Tribes are an early form of social structures
within Eternum and rely on player-driven interactions including arrangements of alliances and declarations of war.
## Formation & Membership
* Any player can create a tribe
* Set tribe name
* Set to public or private
* If private, send invites to player
Flow to create tribe
---
file: docs/pages/eternum/victory.mdx
meta: {}
import { ETERNUM_CONFIG } from "@/utils/config";
import {
HyperstructureInitializationShardsTable,
HyperstructureConstructionCostTable,
HyperstructurePointsTable,
getTotalHyperstructureCompletionPoints,
HYPERSTRUCTURE_POINT_MULTIPLIER,
} from "@/components/HyperstructureTable";
import { formatNumberWithCommas } from "@/utils/formatting";
# 👑 Victory
## Victory Points
Ultimate victory in Eternum is measured by Victory Points (VP), earned exclusively through Hyperstructures. When a
single player’s total VP reaches the season-defined threshold, they unlock the ability to End Season, immediately
halting all further gameplay actions and cementing the final standings. Thus, the race to build, seize, and defend
Hyperstructures is the decisive path that ultimately closes the chapter on each season of Eternum.
## Activating Foundations
Each Hyperstructure is unique, requiring varying quantities of all 22 resources (and Labor) for completion. To activate
a Hyperstructure Foundation, all **{
formatNumberWithCommas(ETERNUM_CONFIG().hyperstructures.hyperstructureInitializationShardsCost.amount)} Ancient
Fragments** must be deposited into the structures's balance, and then the owner of the mine must explicitly activate the
foundation to begin construction. Upon activation, the exact quantity required for each resource is randomly determined
within predefined minimum and maximum ranges shown below:
*Note: In addition to these resources, all Hyperstructures require a fixed contribution of 50,000,000 units of labor to
complete construction.*
## Construction Victory Points
The Lord initiating the Hyperstructure construction determines whether contributions can be made by all Lords (public)
or restricted to members of their tribe (private). Contributions may be made directly from Realms or Villages without
requiring donkeys for transport. As Lords contribute to the Hyperstructure, they are awarded with a proportional
allocation of Victory Points. A total of **{formatNumberWithCommas(getTotalHyperstructureCompletionPoints())}** are
available to claim throughout the construction process.
## Accumulation Victory Points
Separately, and in addition to the victory points awarded at completion, the owner of the Hyperstructure begins to
accumulate
**{formatNumberWithCommas(Number(ETERNUM_CONFIG().hyperstructures.hyperstructurePointsPerCycle)/HYPERSTRUCTURE_POINT_MULTIPLIER)}
victory points** for every second the structure remains under their control. These accumulating victory points can be
shared among multiple Lords through a customizable share split, allowing the owner to distribute glory and reward
cooperation. If a Hyperstructure is claimed by another Lord, future accumulated victory points are redirected to the new
owner, who may similarly decide on sharing arrangements.
## Total Victory
Victory points earned through construction contributions or accumulation cannot be lost once awarded. The first player
to reach
**{formatNumberWithCommas(Number(ETERNUM_CONFIG().hyperstructures.hyperstructurePointsForWin)/HYPERSTRUCTURE_POINT_MULTIPLIER)}
total victory points** unlocks the ability to select the ‘End Season’ option, concluding the current game season.
---
file: docs/pages/eternum/world-physics.mdx
meta: {}
import { Biomes } from "@/components/Biomes";
# 🌪️ World Physics
In Eternum, every action is governed by onchain physics—immutable rules encoded directly onto the blockchain, ensuring
fairness, transparency, and absolute consistency for all Lords. Here, code is law; no exceptions exist outside the
boundaries set by these protocols.
## Seasons
Welcome to Season 1. Eternum, in its current form, comes and goes in seasonal launches. Each season marks a special time
of iteration and advancement in this new form of onchain gaming and may be likened to the movement of tides. Once a
season launches, the tide flows out and the Lords arrive to build their castles in the sand. Nothing, not even the
developers, can stop or alter the course of a season once launched, and players are free to take any action that the
rules of the game allow. Once a Lord accumulates the requisite Victory Points, they unlock the ability to end the
season—signalling the tide to come back in and put an end to the Lords' fun… until next season.
## Time
Time within a season is measured in Eternum Days, and each is precisely one hour in length. One day ends and the next
begins at the top of every real-world hour.
## Materials
In the world of Eternum, everything holds tangible, tokenized value—be it food, labor, resources, troops, or even the
trusty donkeys required for transport. These fungible tokens are generated within Eternum, but can be bridged in and out
of the game through Realms or Banks and traded on external marketplaces. All materials have an associated weight, which
determines the amount that can be stored and the number of donkeys required to move the goods from place to place.
Note: This document will use 'materials' to refer to all tangible assets - i.e. food, resources, ancient fragments,
labor, troops, and donkeys. This is not to be confused with 'resources', which specifically refers to the 22 resources
ranging from wood through to dragonhide. Tokens such as $LORDS and $STRK are not considered materials.
## Cost of Production
With the exception of food, nothing in Eternum is produced for free; every unit of production demands an input—food,
labor, resources, or some combination thereof. Lords must consider these costs and effectively balance trade to ensure
they can survive the season.
## Travel
Transporting materials across the vast lands of Eternum takes time… and donkeys. Every transfer of materials between
locations relies exclusively on these speedy and dependable creatures. These donkeys are single-use, consumed upon
completing their journey, making continuous acquisition vital for maintaining trade routes and strategic flexibility.
Moving tokenized troops through the donkey network may be fast, but to dominate your enemies and ensure security of your
lands, troops must be deployed to an army. Once troops are deployed, they cannot be retokenized, and are doomed to their
fate within this season of Eternum. Once troops are deployed to the world map, their mobility is governed by stamina,
limiting the distance they can travel and the number of actions they can perform. Travelling between hexes or initiating
an attack expends valuable stamina points, but they are partially replenished each Eternum Day.
## Hexes
Eternum unfolds across an infinite, procedurally-generated, hexagonal-tile world map. This represents the physical space
of the world and defines spawn locations, biome distribution, and distances between Realms, Villages, and other world
structures. This is the layer in which armies explore, fight, raid and capture, and is explorable in the 'World' view.
Zooming in, each Realm or Village hex is broken up into smaller hexes which define the local area and building placement
(hexes within hexes!). Here in the 'Local' view, Lords can plan out their building placement and closely manage their
population, supply chain, and production.
## Biomes
Each hexagonal tile on the world map possesses a distinct biome. Each troop type responds uniquely to these varied
terrains, gaining or losing combat efficiency and stamina based on their affinity with different biomes. Successful
Lords must master biome knowledge to exploit strategic advantages or avoid costly missteps.
## The Known World
Initial scouting reports have revealed some key details about the world:
* Six ancient Banks have been located, distributed evenly in a ring around the central point. These structures are
perfectly situated and designed to facilitate trade in this new world. Sufficiently prepared Lords may be able to
capture these lucrative structures, but will likely make themselves a target in the process.
* An unknown number of Hyperstructure Foundations are scattered around the unexplored world. These are rare, key
locations that must be claimed by Lords in order to rebuild the ancient Hyperstructures that are critical to achieving
victory. They are more likely to be found in the central regions of the map, and as each subsequent Hyperstructure
Foundation is discovered, the likelihood of finding another one in the world diminishes.
* Hundreds of mines are also strewn across the landscape. Prospectors have theorized that these mines are hotspots for
finding ancient fragments needed to reconstruct key sections of the Hyperstructures.
* Bandits roam throughout the lands and have established lairs in each of the key locations described above. The newly
arrived Lords will need to overcome these lawless brigands to bring order and control back to Eternum.
* Mysterious hooded figures lurk in the mist, offering to play a strange card game with any who wish to try their luck.
---
file: docs/pages/eternum/world-structures.mdx
meta: {}
# 💠 World Structures
## Hyperstructures
Hyperstructures are colossal edifices constructed atop ancient foundations hidden across Eternum, representing
monumental achievements and symbols of dominance in the game world. Lords must uncover and claim Hyperstructure
Foundations through exploration and combat, then invest substantial quantities of resources, labor, and ancient
fragments to reconstruct these majestic towers. Successfully completing and controlling Hyperstructures is the primary
path to victory in Eternum, making them ultimate strategic targets for ambitious Lords and powerful tribes alike.
### Hyperstructure Foundations
These ancient, indestructible platforms are primarily scattered within the ring of Banks that surround the centre of the
map. There is a chance of discovering a Hyperstructure Foundation each time an army explores an unrevealed hex,
calculated using the following formula:
```
Discovery Chance = (0.975^x × y) - (z × 0.001)
```
Where:
* **x** = the number of hexes from the centre of the map
* **y** = the base chance of finding a Hyperstructure Foundation on the central hex (4%)
* **z** = the number of Hyperstructure Foundations already discovered
For example, if you're exploring a hex 100 tiles from the center and 5 foundations have already been discovered:
```
Discovery Chance = (0.975^100 × 4) - (5 × 0.001)
= (0.079 × 4) - 0.005
= 0.316 - 0.005
= 0.311 or 31.1%
```
The result of this function means that Hyperstructure Foundations are generally only able to be found within 300 tiles
of the centre of the map. As more are found, the chance of finding another diminishes and makes it even less likely to
find one further from the centre. Lords are therefore incentivised to explore early in the season to discover these key
components for victory.
Hyperstructure Foundations and Hyperstructures both have four defense slots to deter attacks from rival Lords. However,
like other world structures they are not able to maintain any field armies.
## Fragment Mines
Fragment Mines are rare, ancient nodes scattered throughout the unexplored hexes of Eternum. They produce valuable
ancient fragments, a vital resource needed for constructing Hyperstructures. Once discovered by an exploring field army,
a Fragment Mine must first be claimed by defeating its resident bandit forces. Only then can Lords begin extracting the
mysterious shards hidden within. Each mine contains a finite number of ancient fragments and will permanently cease
production when depleted.
The chance of discovering a Fragment Mine when exploring an unrevealed hex is static at 1 in 150, or 0.67%. Mines can
contain between 300,000 and 3 million ancient fragments in incremental amounts (i.e. 300k, 600k, 900k…). It will take
some time for the full amount of ancient fragments to be produced by the structure, and the more that the mine contains,
the longer it will take. Lords will need to ensure that their mines are adequately defended from rivals, however they
only have one defense slot to support a guard army.
---
file: docs/pages/overview/controller.mdx
meta: {}
import { importantNote } from "@/components/styles";
***
title: Cartridge Controller description: Using the Cartridge Controller in the Realmverse
***
# Cartridge Controller
The Cartridge Controller is a gaming-focused smart contract wallet that makes Web3 gaming accessible and transactionless
via Session Keys. You must have a controller to play Eternum or Blitz. To find out more about the controller, read their
documentation [here](https://docs.cartridge.gg/controller/overview).
## Using the Controller
If you are playing with a Village in Eternum or entering a Recruit game in Blitz, you can play purely using the
controller. However, to use Season Passes and **$LORDS**, you will need to transfer them to your controller from
Starknet using your ArgentX or Braavos wallet.
To use the controller, you can either log in or sign up when starting either Eternum or Blitz. To create a controller,
simply input a username and sign up.
There are multiple ways to securely sign up:
* **Passkey**: Passkeys are a modern and secure way to authenticate users without passwords. They use public key
cryptography and are stored by platform authenticators such as Face ID, Touch ID, or password managers like Bitwarden
or 1Password.
* **Metamask**: Connect using your MetaMask wallet.
* **Rabby**: Connect using your Rabby wallet.
* **Discord**: Connect using your Discord account.
* **Wallet Connect**: Connect using a wide range of wallets.
⚠️ IMPORTANT NOTE
If you are choosing Passkey on a Windows PC that does not have Bluetooth functionality, you will need to use a
password manager to set up your controller. There is a known issue in which Windows will ask for a Windows Hello or
external security key (USB) that wasn't used in the controller setup, preventing you from being able to sign in.
Once this is complete, you will be able to 'Create Session'. This enables all of the game functions in a transactionless
session.
### Transfer Season Pass to Controller
Transfer an Eternum season pass to your controller easily [here](https://empire.realms.world/season-passes). Connect
your controller by selecting the controller icon located at the top of the page and select the passes to transfer.
## Controller Key Features
### Simple and Secure
* Passwordless authentication using Passkeys for one-click onboarding
* Self-custodial embedded wallets that put players in control
* Built-in security features to protect player assets
### Designed for Seamless Gameplay:
* Session keys eliminate transaction popups during gameplay
* Secure transaction delegation lets games submit actions on behalf of players
* Free transactions through the Cartridge Paymaster so players focus on playing
### Supported Platforms and Passkey Backup
Passkeys are well-supported across modern platforms. You can use them with device authenticators directly or pair them
with a mobile device using the QR flow. For those without device authenticators, several password managers like
Bitwarden (free), 1Password, and Dashlane support Passkeys.
#### Backing Up Your Passkey
* Apple devices: Passkeys are backed up along with your keychain in iCloud.
* Android devices: Passkeys are backed up with your Google account.
* Windows devices: Passkeys are created and managed as part of your Windows account.
#### Using Controller Across Devices
Currently, Controller availability across devices depends on how the Passkey was created. Some platforms automatically
synchronize them across devices for seamless use. For cross-platform usage (e.g., Apple and Android), synchronization
might not be available. For now, we recommend using a Password Manager or the QR flow to support this configuration.
Multi-credential support is coming soon to enhance device compatibility.
Should you have any issues with the controller or require support, you can join the Cartridge Discord
[here](https://discord.gg/cartridge).
---
file: docs/pages/overview/disclaimer.mdx
meta: {}
import { formatNumberWithSpaces } from "@/utils/formatting";
import { ETERNUM_CONFIG } from "@/utils/config";
import { Callout } from "vocs/components";
import { HYPERSTRUCTURE_POINT_MULTIPLIER } from "@/components/HyperstructureTable";
# Disclaimer and Risk Acknowledgment
Important Disclaimer – Please Read Carefully
By participating in Realms games, you fully acknowledge and accept the following terms and conditions.
## Immutable Contracts
Each game is governed entirely by **immutable** smart contracts. Once deployed, the game's rules and mechanics **cannot
be altered, updated, or reversed** by the developers, the DAO, or any other party. These smart contracts have **not**
been audited. Players should fully understand the implications of interacting with the system and assess the risks
involved.
## Risk of Loss
All transactions and gameplay actions in these games are **final**. There are no mechanisms for refunds, reversals, or
compensation. You acknowledge the **risk of loss of funds** and accept that you bear sole responsibility for any
financial impact incurred.
## Eternum
A season of Eternum concludes when a single player achieves the required
**{formatNumberWithSpaces(Number(ETERNUM_CONFIG().hyperstructures.hyperstructurePointsForWin)/HYPERSTRUCTURE_POINT_MULTIPLIER)}**
Victory Points and clicks the "End Season" button. At this point:
### ⚠️ SEASON END CRITICAL INFORMATION
When the season ends, **ALL in-game actions are DISABLED** except:
* transfer materials
* deposit resource arrivals
* retrieve LP positions
* cancel market orders
* bridge materials out of the game
1. **7-Days Withdrawal Window (starts immediately when "End Season" is clicked)**
* Starting from the exact moment the season ends, you have **7 days** to bridge out **ALL $LORDS** tokens and
bridgeable materials from your Realms' balance
2. **After 7 Days**
* Any remaining **$LORDS** tokens and **Materials** will be **permanently locked**
* These assets **CANNOT** be recovered under any circumstances
3. **Score Registration**
* Players have **4 days** to register their scores
* Registration is **MANDATORY** to be eligible for prizes
* While registration has a deadline, prize claiming does not
Please refer to the documentation for detailed instructions on the bridging process during active gameplay.
## Blitz
Blitz game instances operate on a fixed schedule with immediate prize distribution upon completion. Unlike Eternum
seasons, Blitz games do not have extended withdrawal windows or bridging requirements, as all gameplay concludes within
the session timeframe.
## No Recourse
By participating in these games, you waive all rights to claims or recourse against the developers, the DAO, or any
other associated entities for any losses or disputes arising from your participation in the game.
## Acknowledgment of Terms
Participation in Realms games constitutes your agreement to these terms, as well as all other terms and conditions
outlined in the game's documentation.
---
file: docs/pages/overview/entry.mdx
meta: {
"title": "Game Entry & Participation",
"description": "How to join and participate in the Realmverse"
}
# Game Entry & Participation
## Eternum
Eternum takes place in 'Seasons', multi-week campaigns with long-term strategic and diplomatic developments. There are
several weeks in between each season that allow for iterative development and feature expansion in each subsequent
season.
### Realms NFT Holder Entry
Realms NFT holders have the exclusive benefit of free entry into each season of Eternum through the minting of Season
Passes. Each Season Pass is unique to the Realm it was minted from and allows the holder to initiate a settling
transaction to enter the game. To do this, Lords must first mint the Season Pass
[here](https://empire.realms.world/mint) using the wallet that contains the Realms NFT, then transfer the Season Pass to
their [Cartridge Wallet](/overview/controller).
### Non-Realm Holder Entry
⚖️ **Season Pass Marketplace**
Should a Realm NFT holder decide not to use their Season Pass, they can transfer it or sell it on the
[Season Pass Marketplace](https://empire.realms.world/trade), providing an avenue for non-Realm holders to acquire
Season Passes to participate in the game.
🏕️ **Villages**
Villages are modest outposts that can be established around settled Realms, offering an accessible entry point into
Eternum. Villages have some restrictions, but offer a low-cost, casual taste of Eternum gameplay to newcomers while also
acting as an avenue for established players to expand their production. Each Realm can support up to six surrounding
Villages.
For detailed information about Villages, including settling mechanics, resource production, and progression paths, visit
our [Villages guide](/eternum/realm-and-villages/villages).
### Realm Control Mechanics
As long as you maintain control of a settled Realm or Village, you can actively participate without additional entry
fees, engage in the market using **$LORDS** tokens, and enjoy uninterrupted gameplay until the end of the season.
However, Eternum is a high-stakes game, it can be unforgiving and players must accept that their valuable holdings may
become a target for other players. Thankfully, the game offers high-stakes and low-stakes gameplay to ensure that
players can participate in the world at their own pace and risk tolerance.
⚔️ **Realms - High Stakes**
Once settled, Realms can be conquered and claimed within a Season, transferring control to the conqueror. Realms are the
key playing pieces in Eternum and allow for the full gameplay experience in producing resources, training armies,
conducting trade, and pursuing territorial expansion. This level of capability comes with increased risk, as Realms must
continually manage defenses, alliances, and strategic positioning to protect themselves from rivals.
🌴 **Villages - Low Stakes**
Unlike Realms, Villages cannot be conquered by other players, making them an ideal choice for casual players seeking a
lower-risk gameplay experience. Villages can still participate in most gameplay activities but production rates are
limited to 50% the rate of Realms and progression paths are limited. These features make Villages perfect for new
players looking to learn the game mechanics without the constant threat of conquest.
## Blitz
While an Eternum season is a single game instance that unfolds over weeks, Blitz competitions take place in 'series'
that are hosted in the development period between seasons. Unlike Eternum, Blitz does not require ownership of Realms
NFTs or Season Passes for entry. Entry requirements and stakes are determined by the bracket that players choose to
compete in.
### Bracket System
Blitz gameplay is structured around three progressively competitive brackets: Recruit, Warrior, and Elite. Each tier
escalates both the stakes and the rewards, catering to various playstyles and competitiveness levels.
🥉 **Recruit - Low Stakes**
Free-to-enter, this bracket is not beholden to being part of a series and serves as an introduction and practice
environment. Players can experience Blitz gameplay with no stakes while potentially earning some low-value Loot Chest
rewards. Recruit bracket games run every day on a varying timetable to encourage participation from players in all time
zones.
🥈 **Warrior - Medium Stakes**
This bracket makes up the bulk of competitive games in a Blitz series. Each Warrior game requires an entry fee in
$LORDS, which contributes to a shared prize pool-bolstered by additional Loot Chest rewards and entry tickets to the
coveted Elite bracket games. Warrior bracket games run a few times a week while the series is active, typically during
prime time gaming windows for three key timezones (USA, Europe, and Asia-Pacific).
🥇 **Elite - High Stakes**
Representing the highest level of Blitz gameplay, Elite bracket games are considered the 'finals' of a Blitz series.
These games don't require an entry fee in $LORDS, instead demanding an entry ticket NFT that can only be earned in
leaderboard positions of Warrior bracket games (or purchased off the secondary market from another Lord). These games
run at the end of a Blitz series and offer substantial $LORDS prize pools, Loot Chest rewards, and prestige to the best
performing Lords.
### Realm Control Mechanics
Each Lord starts the game in control of three equally capable Realms. As in Eternum, Realms in Blitz can be conquered by
other Lords, encouraging constant vigilance and strategic interaction. Lords must carefully balance their military
forces, territorial positioning, and resource management to survive and thrive within the intense two-hour game
duration. There is no progression beyond the conclusion of the game and each game instance starts with a refreshed world
state.
---
file: docs/pages/overview/introduction.mdx
meta: {
"title": "Introduction to the Realmverse",
"description": "Understanding the Realmverse"
}
# Welcome to the Realms
## The Lords Have Arrived
Across treacherous open seas and endless horizons, the legendary Galleon has finally arrived in a new land, bearing the
brave and ambitious souls known as Lords. Having journeyed for years, driven by dreams of power, glory, and untold
riches, these Lords have finally set foot upon their destination—a mysterious landscape ripe with ancient secrets and
unclaimed territories. Now, as the mists clear, each Lord must forge their own path in this land of promise and peril.
## Establish Your Foothold
Your task begins by settling a seat of power from which your destiny unfolds. Find your place upon the vast, unexplored,
procedurally generated world map and secure vital materials to fuel your expansion. Grow your influence through shrewd
economic choices, military strategy, and diplomatic maneuvering, nurturing your humble settlement into a mighty empire.
Yet, strength alone is insufficient, for Eternum is fraught with dangers. Bandits roam unchecked and rival Lords covet
your lands. Armies must be raised, resources guarded, and alliances carefully chosen. Only through wise leadership and
vigilant defense can your holdings flourish and endure.
## Find Your Glory
These new lands are dominated by colossal relics from an ancient civilization, known as Hyperstructures. The mysterious
towers act as conduits of power, providing their masters with the ability to assert dominance over the continent and
their subjects. They are the focus of ultimate victory.
However, this new and expansive world offers glory beyond mere conquest; whether through trade mastery, military
prowess, diplomatic excellence, or simply the joy of exploration, every Lord has the freedom to find their own path to
greatness.
## Core Pillars
* **Fully Onchain Gameplay** - Every action, decision, and outcome is transparently executed and verifiable onchain.
Secured by Ethereum and seamlessly scaled by Starknet, the integrity and immutability of gameplay are guaranteed.
* **Player‑Driven Economy** – Every resource produced, trade executed, and asset exchanged originates solely from player
decisions. Collectively, these choices dictate supply, demand, and market prices, forming a dynamic and ever-evolving
economy across the entire ecosystem.
* **Strategic Depth** – Long-term success requires efficient production management, tactical territorial expansion, and
precisely timed military engagements. Lords who exhibit foresight, adaptability, and strategic mastery will rise to
dominate, while others fall into obscurity.
## Game Modes
Lords can test their skills and strategies in two distinct game modes, each offering unique experiences and challenges:
**Eternum** - The flagship seasonal game mode, Eternum invites players into a rich, evolving world of strategic depth.
Unfolding over several weeks, Lords shape the world through expansive empire-building, intricate economic management,
diplomatic maneuvering, and focused military campaigns. The complexity of Eternum is balanced by accessible gameplay
layers, allowing casual players to participate in lower-stakes activities such as resource trading, exploration, and
minigames. Whether aspiring to dominate the continent or simply carving out a peaceful corner of the world, Eternum
offers rewarding experiences suited to every play style.
**Blitz** - Blitz provides an exciting contrast through rapid, high-intensity, free-for-all competition lasting exactly
two hours. This mode distills strategic gameplay into streamlined mechanics, accelerated resource production, and
immediate military engagements, rewarding quick thinking and decisive action. Blitz is structured around a three-tier
competitive bracket system—Recruit, Warrior, and Elite—allowing Lords to engage at their preferred intensity level, from
casual challenges to elite competitions with significant stakes and rewards. Whether testing new strategies or vying for
glory, Blitz delivers exhilarating, accessible, and fiercely competitive battles.
## Rewards
Lords who prove their skill and strategic prowess across both Eternum and Blitz are rewarded generously for their
efforts. Success in competitive events can award portions of [$LORDS token](/overview/lords) prize pools, as well as
[Loot Chests](/overview/loot-chests) containing consumables and exclusive cosmetic items.
## Vision
Explore our
[detailed vision document](https://github.com/BibliothecaDAO/world-guide/blob/main/realms-world-guide-v1.0.0.pdf)
---
file: docs/pages/overview/key-concepts.mdx
meta: {
"title": "Key Concepts",
"description": "The foundational mechanics of Eternum"
}
# 🔑 Key Concepts
This section outlines the core mechanics and systems that form the foundation of Eternum's gameplay.
## Realms
* **Limited Assets**: 8,000 unique and irreplaceable Realms serve as the foundation of Eternum, each has the ability to
freely mint a Season Pass.
* **Settling**: Players must burn a Season Pass to settle their Realm in the game world.
* **Starting Materials**: Each newly settled Realm begins with a set of starting materials.
* **Progression Path**: Realms can upgrade from Settlements → Cities → Kingdoms → Empires, unlocking additional
buildable hexes and defensive army slots.
* **Resource Production**: Each Realm can produce 1-7 different resources based on the original Realms NFT metadata.
* **Wonders**: Realms with Wonders have a 20% production bonus and share this bonus with all Realms and Villages in a 12
tile radius.
* **Hardcore Gameplay**: If a Realm’s defenses crumble, it can be claimed by another player—transferring gameplay rights
to the conqueror.
## Villages
* **Accessible Entry**: Villages can be established around settled Realms, offering a simple entry system for new
players
* **Scaling**: Up to 6 Villages per Realm, allowing for 48,000 Villages in Season 1
* **Limited Production**: Produce materials at 50% the rate of Realms and only have access to 1 resource type each
* **Parent Realm**: Each Village is tied to a parent Realm and will pay bridging fees if moving materials out of the
game
* **Troop Restrictions**: Villages may only receive tokenized troops from their parent Realm, they cannot buy or be sent
troops from any other source
* **Simple Progression**: Villages have a limited upgrade path from Settlement to City
* **Casual Gameplay**: Villages can be raided, but can’t be claimed by other players.
## Materials
* **Materials Categories**:
* **Food**: (Wheat, Fish) - essential for production, troop movement, and construction.
* **Resources**: 22 different resource types, distributed based on rarity across both Realms and Villages.
* **Troops**: Units with unique properties that can be assigned to armies.
* **Donkeys**: Required for moving materials around the world, essential for logistics.
* **Labor**: A new material in S1, allows players to build and produce in 'simple mode', acquired through burning
resources.
* **Ancient Fragments**: Found in game, critical for the construction of Hyperstructures.
* **ERC20 Compatibility**: All materials (except Labor) can be traded freely and bridged out as ERC20 tokens through
Realms.
## Buildings
* **Construction Modes**: Two building systems available - Standard (resource-efficient) and Simple (labor-focused).
* **Building Types**:
* **The Keep**: Central structure automatically constructed when settling, provides baseline population capacity.
* **Resource Buildings**: Specific buildings for each of the 22 resources, only requiring food to construct.
* **Economic Buildings**: Includes Farms, Fishing Villages, Worker Huts, Storehouses, and Markets.
* **Military Buildings**: Nine different buildings for producing three tiers of each troop type.
* **Placement & Population**: Buildings require buildable hexes and population capacity, with more unlocked as Realms
and Villages are upgraded.
## Production
* **Production Modes**: Like buildings, production can be done in Standard (resource-intensive) or Simple (food and
labor) mode.
* **Production Types**:
* **Food**: The only resources (Wheat, Fish) that can be produced without inputs.
* **Resources**: Require combinations of other resources (standard) or labor (simple).
* **Labor**: Produced by burning resources in the Keep, used for simple mode construction and production.
* **Troops**: Essential for exploration and warfare, with higher tiers requiring lower tier troops as inputs.
* **Donkeys**: One-time-use transport, consumed after a single journey.
## World Map
* **Exploration System**: The world begins shrouded in mystery, with only the six Banks visible at season start;
adjacent hexes are revealed when settling, and armies can explore to reveal new areas.
* **Biomes**: 16 unique biome types procedurally generated across the map, each affecting combat effectiveness and troop
movement.
* **Movement & Stamina**: Armies move using stamina (regenerating at +20 per Eternum Day), with costs varying by biome
and troop type; Knights, Crossbowmen, and Paladins each have different maximum stamina capacities.
* **Exploration Mechanics**: Exploring costs 30 stamina per hex and reveals that area permanently to all Lords;
exploring armies may discover resources or encounter world structures and agent armies.
## Military
* **Army Composition**: Armies consist of a single troop type and tier (Knights, Crossbowmen, or Paladins).
* **Troop Tiers**: T1, T2, and T3 troops with increasing combat strength.
* **Combat Factors**: Battles resolved based on army damage, stamina modifiers, and biome effects.
* **Army Types**:
* **Field Armies**: Deploy onto the world map for exploration and conquest.
* **Guard Armies**: Deployed to defense slots to protect structures.
* **Stamina System**: Armies recover +20 stamina daily, with 30+ stamina needed for full combat effectiveness.
* **Biome Effects**: Each troop type has advantages (+30% damage), disadvantages (-30% damage), or no change (0%) in
specific biomes.
* **Raiding Mechanics**: Armies can attempt to steal resources from structures without defeating defenders.
* Success depends on the raiding army's damage relative to the combined guard armies.
* Undefended structures can be raided without casualties.
## Tribes
Tribes are player-formed organizations enabling cooperation and coordination. They can be made public (open to all) or
private (invitation only) and allow players access to tribe-only features. Tribes are an early form of social structures
within Eternum and rely on player-driven interactions including arrangements of alliances and declarations of war.
## Victory Conditions
Eternum's seasonal gameplay culminates in victory through the accumulation of victory points earned through the
construction and ownership of Hyperstructures. However, there are various achievement paths, with players earning
recognition and rewards for various actions within the Season.
---
file: docs/pages/overview/links.mdx
meta: {
"title": "Quick Links",
"description": "Essential links for Eternum"
}
# Quick Links
## Play & Learn
* [Empire Home](https://empire.realms.world/) - The home of Realms games
* [Play Eternum](https://eternum.realms.world/) - Season 1 has concluded
* [Realms NFT Marketplace](https://empire.realms.world/trade/realms) - Trade your Realms NFTs
* [Loot Chest Marketplace](https://empire.realms.world/trade/loot-chests) - Trade Loot Chests
* [Statistics](https://stats.eternum.realms.world/) - Revenue and gameplay stats
* [GitHub](https://github.com/BibliothecaDAO/eternum) - Explore the code
## Community
* [Realms World](https://realms.world/) - The DAO behind Eternum
* [Discord](https://discord.gg/realmsworld) - Join our discord
* [Twitter](https://x.com/LootRealms) - Follow the community
* [Twitter](https://x.com/RealmsEternum) - Follow for Realms game updates
---
file: docs/pages/overview/loot-chests.mdx
meta: {
"title": "Loot Chests",
"description": "Understanding Loot Chests in the Realmverse"
}
import { importantNote } from "../../components/styles";
# 🎁 Loot Chests
Loot Chests are reward containers that Lords can earn through various activities in the ecosystem. These chests are
primarily rewarded for achievements and high-scoring gameplay, and are rumoured to contain advantageous consumables or
flashy cosmetic items.
The secrets of the Loot Chests are yet to be revealed, as they are not yet able to be opened. But some Lords are already
speculating on the contents of Eternum Rewards Chests from Season 1 on the
[Loot Chest Marketplace](https://empire.realms.world/trade/loot-chests).
## Epochs
Epochs mark the distinct eras of gameplay and development. An epoch begins with the start of each new Eternum season and
ends with the start of the next. Each Loot Chest carries the mark of its epoch, defining not only when it was earned but
also the specific rewards contained within. Chests from different epochs therefore maintain their unique character over
time, and can be opened or left sealed at the owner's discretion (as some Lords may wish to speculate on long-term
sealed chest value).
## Chest Types in Epoch 1
The current epoch introduces four distinct chest types with varying contents, each obtained through different gameplay
experiences within the ecosystem.
**Eternum Rewards Chest** - These premium chests are earned through participation and success in the flagship seasonal
game mode. 2,244 Eternum Rewards Chests were distributed to players of Eternum Season 1 on the basis of their
achievement score. There will be no further issuance of these chests in Epoch 1.
**Blitz Rewards Chest** - Fast-paced competition in Series 1 Blitz games will yield these chests. (Warrior and Elite
bracket only)
**Elite Blitz Rewards Chest** - The highest tier of Blitz competition produces these exclusive chests, reserved for
Lords who prove themselves in Elite bracket games.
**Relic Chest** - These mysterious containers hold various Relics, consumables that can give Lords an edge in
competition when used correctly. They can be earned through participation in any Blitz bracket and through other
yet-to-be-announced interactions in the ecosystem.
⚠️ IMPORTANT NOTE
Loot Chests are a feature in development. Keep an eye out for announcements in the Realms Discord relating to Loot
Chest rewards. This page will be updated as more information is made available to the community.
---
file: docs/pages/overview/lords.mdx
meta: {}
# $LORDS Token
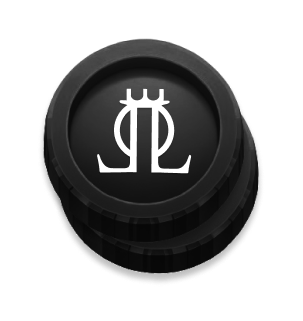
$LORDS is the native token of the Realms Autonomous World. It serves as the primary currency within the ecosystem,
enabling economic activity and participation in various games.
## Token Details
* **Name**: LORDS
* **Network**: Starknet
* **Contract Address**:
[0x0124aeb495b947201f5fac96fd1138e326ad86195b98df6dec9009158a533b49](https://starkscan.co/token/0x0124aeb495b947201f5fac96fd1138e326ad86195b98df6dec9009158a533b49)
* **Total Supply**: 300,000,000 LORDS
## $LORDS in Gameplay
* **Trading Currency**: $LORDS serves as the primary currency for trading within Eternum's Automated Market Maker (AMM)
and the peer-to-peer Orderbook, allowing Lords to facilitate trades and manage their economic interactions.
* **Transport System**: $LORDS are essential for producing donkeys, the backbone of Eternum's transport system,
effectively acting as the "gas" token required for facilitating all in-game logistics and resource transfers.
* **Blitz Entry Fees**: In Blitz series, $LORDS are used for entry fees in Warrior bracket competitions, with these fees
contributing to the prize pools distributed to top performers.
## How to get $LORDS
### In Game
Within Eternum, Lords can obtain $LORDS through various methods:
* Selling surplus materials through the in-game AMM or Orderbook
* Defeating or negotiating with AI agents
* Discovering and playing minigames scattered throughout the Eternum world
* Earning fees on AMM trades by controlling a Bank
* Playing the game and receiving allocations from various prize pools
Players can also earn $LORDS by winning Blitz tournaments and earning prize pool distributions.
### On Starknet
Outside of gameplay, $LORDS can be acquired on the Starknet network through decentralized exchanges (DEXs).
#### Recommended Exchanges
| Exchange | Compatibility | Notes |
| --------------------------------------------------------------------------------- | --------------------------------- | --------------------------------------------------------------------------------------------------------------------------------- |
| [Ekubo](https://app.ekubo.org/?outputCurrency=LORDS\&amount=1\&inputCurrency=ETH) | ✅ Supports Cartridge Controller | Swap directly with your game wallet |
| [Avnu](https://app.avnu.fi/en/eth-lords) | ❌ No Cartridge Controller support | Requires an [Argent](https://x.com/argentHQ) or [Braavos](https://x.com/myBraavos) wallet first, then transfer to your controller |
#### Step-by-Step Guide
For new users, follow this [comprehensive guide](https://x.com/lordcumberlord/status/1920650310621495350) that covers:
1. Setting up a Braavos wallet
2. Bridging ETH to Starknet via Orbiter
3. Swapping ETH for STRK and $LORDS
4. Creating a Cartridge Controller (if needed)
5. Transferring $LORDS to your game wallet
---
file: docs/pages/overview/resource-addresses.mdx
meta: {}
import { ResourceAddresses } from "@/components/ResourceAddresses";
# Resource Addresses
---
file: docs/pages/blitz/materials/automation.mdx
meta: {}
# 🤖 Resource Automation
Resource automation allows players to set up continuous production processes and automated transfers within their realms
and between entities. This system helps manage resource generation and distribution efficiently without constant manual
intervention.
## Overview
The automation system works by processing two types of "orders" that you define:
1. **Production Orders**: Automate resource production within your realms
2. **Transfer Orders**: Automate resource transfers between your entities (realms, villages, hyperstructures, etc.)
Both types of orders specify what you want to automate, the method or conditions, target amounts or schedules, and
priorities.
**Key Features:**
* **Order-Based**: Define specific production and transfer tasks.
* **Priority System**: Control the sequence of execution for multiple orders.
* **Multiple Automation Types**: Choose between production automation and transfer automation.
* **Entity-Specific**: Automation is configured on a per-entity basis.
* **Browser Dependent**: Automation processes run in your browser; the game tab must remain open for automation to
function.
## How It Works
The automation system periodically checks and processes active orders for each entity.
1. **Processing Interval**: All orders are evaluated every 10 minutes.
2. **Order Prioritization**: For each entity, orders are sorted by their priority number (1 being the highest).
3. **Pause Functionality**: You can pause all automation for any entity, which will skip all orders for that entity.
4. **Order Processing**: The system also processes different order types.
### Production Order Processing
* **Balance Check**: Before executing, the system checks if the realm has sufficient input resources or labor.
* **Recipe-Based Production**: Production follows predefined recipes from the game's configuration.
* **Cycle Calculation**: Determines the maximum number of production cycles possible based on available inputs.
* **Transaction**: If conditions are met, a blockchain transaction is initiated to perform the production.
### Transfer Order Processing
* **Schedule Check**: For recurring transfers, checks if enough time has passed since the last transfer.
* **Threshold Check**: For conditional transfers, checks if resource levels meet the specified conditions.
* **Resource Availability**: Verifies the source entity has sufficient resources for the transfer.
* **Transaction**: If conditions are met, initiates a transfer transaction between entities.
## Production Types
There are three types of production automation:
1. **Standard (Resource-to-Resource)**:
* Converts one or more input resources into a different output resource.
* Example: Using Wood and Stone to produce Tools.
2. **Simple (Labor-to-Resource)**:
* Utilizes Labor from the realm to produce a specific resource.
* Example: Using Labor to harvest Food.
3. **Resource-to-Labor**:
* Converts a specified resource into Labor for the realm.
* Example: Burning Food to generate Labor.
## Production Order Modes
Production orders can operate in two different modes:
1. **Produce Once**:
* Produces resources until the target amount is reached, then stops.
* Shows progress as "Produced / Target" (e.g., "1,500 / 5,000").
* Can be set to "Infinite" to produce continuously without a limit.
2. **Maintain Balance**:
* Keeps resource balance at the target level.
* Production triggers when balance drops below target minus buffer percentage.
* Example: Target 10,000 with 10% buffer = production starts when balance drops below 9,000.
* Useful for maintaining steady resource levels.
## Transfer Types
Transfer automation allows you to automatically move resources between your entities. There are three transfer modes:
1. **Recurring Transfers**:
* Transfers resources at regular intervals.
* Minimum interval: 10 minutes (due to automation cycle).
* Example: Transfer 1,000 Food from Realm A to Realm B every 2 hours.
2. **Maintain Stock Transfers**:
* Transfers when the destination entity falls below a threshold.
* Example: Transfer 500 Wood to Realm B when it has less than 1,000 Wood.
* Helps keep important entities well-supplied.
3. **Depletion Transfers**:
* Transfers when the source entity exceeds a threshold.
* Example: Transfer excess Stone when Realm has more than 5,000 Stone.
* Prevents resource overflow and distributes surplus.
## Managing Automation Orders (UI)
You can manage automation orders through the "Automation" tab within any entity's interface, or through the global "All
Automations" view.
### Production Automation
Production automation is managed within individual realm interfaces.
#### Adding a New Production Order
1. Click the "Add New Automation" button.
2. Configure the following:
* **Order Mode**:
* `Produce Once`: Produce up to target amount then stop
* `Maintain Balance`: Keep resource balance at target level
* **Production Type**:
* `Standard (Resource-based)`
* `Simple (Labor-based)`
* `Resource to Labor`
* **Priority (1-9)**:
* Set the execution priority. `1` is the highest priority, `9` is the lowest.
* **Resource to Produce / Resource Input for Labor**:
* Select the resource you want to produce or consume.
* The UI shows required input resources for Resource-to-Resource recipes.
* **Target Amount**:
* For "Produce Once": Set total quantity to produce, or check "Infinite" for continuous production.
* For "Maintain Balance": Set the balance level to maintain.
* **Buffer Percentage** (Maintain Balance only):
* Production starts when balance drops below target minus buffer percentage.
3. Click "Add Automation".
### Transfer Automation
Transfer automation allows you to move resources between any of your entities.
#### Adding a New Transfer Order
1. **Select Source Entity**:
* Choose entity type (Your Realms, Your Villages, etc.)
* Search and select the specific source entity
2. **Configure Transfer**:
* **Transfer Mode**:
* `Recurring`: Transfer at regular intervals
* `Maintain Stock`: Transfer when destination is low
* `Depletion Transfer`: Transfer when source is high
* **Destination Entity**:
* Choose entity type and select specific destination
* **Resources to Transfer**:
* Add multiple resources with specific amounts
* **Schedule/Conditions**:
* For Recurring: Set interval in minutes (minimum 10 minutes)
* For conditional transfers: Set threshold amounts
3. Click "Add Transfer Automation".
#### Transfer Interval Guidelines
* **Minimum Interval**: TBC (matches automation processing cycle)
* **Custom Intervals**: Custom intervals can be set in minutes
* **Effective Timing**: Transfers occur when automation cycles align with your interval
### Viewing and Managing Orders
#### Individual Entity View
Each entity shows its automation orders in a table with:
* **Priority**: Order execution priority
* **Mode**: "Once", "Maintain", or transfer mode ("Recurring", "Stock", "Depletion")
* **Resource**: Visual representation of resource flow
* **Target/Balance**: Target amounts or transfer schedules
* **Produced**: Amount produced (production orders only)
* **Type**: Order type (production or transfer)
* **Actions**: Remove button
#### Global Automation View
The "All Automations" view shows orders from all entities:
* **Realm Filter**: Filter by specific realm or view all
* **Order Status**: Visual indicators for completed, paused, or active orders
* **Countdown Timer**: Shows time until next automation cycle
* **Bulk Actions**: Pause/resume or delete orders
### Pausing Automation
You can pause automation at different levels:
1. **Individual Entity**: Pause all automation for a specific entity
2. **Individual Order**: Remove specific orders
3. **Global Control**: Manage all orders from the global view
## Important Considerations
### General Requirements
* **Browser Must Be Open**: Automation processing occurs in your browser. If you close the game tab or your browser,
automated processes will stop.
* **10-Minute Processing Cycle**: All automation is processed every 10 minutes. This affects timing for all orders.
* **Transaction Costs**: Each successful automation cycle that results in production or transfer will involve a
blockchain transaction, which incurs network transaction fees.
* **Processing Time**: Due to the 10-minute processing interval and potential network conditions, there might be a delay
before an order is executed or its results are visible.
### Production Automation Considerations
* **Resource Availability**: Production orders will only execute if the required input resources are available in the
realm at the time of processing.
* **Recipe Dependencies**: Production follows predefined game recipes. You cannot produce resources that don't have
valid recipes.
* **Storage Limits**: Produced resources will increase your realm's balance and may cause resource loss if storage is
full.
### Transfer Automation Considerations
* **Entity Ownership**: You can only set up transfers between entities you own.
* **Transfer Timing**:
* Minimum effective interval is 10 minutes due to processing cycle
* Intervals shorter than 10 minutes will effectively transfer every 10 minutes
* Non-10-minute multiples may have irregular timing based on when transfers align with processing cycles
* **Resource Availability**: Transfers will only execute if the source entity has sufficient resources at processing
time.
* **Multiple Resources**: You can transfer multiple different resources in a single transfer order.
* **Conditional Logic**: Maintain Stock and Depletion transfers check conditions each cycle and only transfer when
thresholds are met.
### Best Practices
1. **Start Simple**: Begin with basic production orders or simple recurring transfers to understand the system.
2. **Monitor Initially**: Watch your first few automation cycles to ensure orders work as expected.
3. **Use Priorities**: Set appropriate priorities when you have multiple orders to control execution sequence.
4. **Plan for Delays**: Account for the 10-minute processing cycle when planning time-sensitive operations.
5. **Check Resource Flows**: Regularly verify that your automated transfers are maintaining desired resource
distributions.
---
file: docs/pages/blitz/materials/bridging.mdx
meta: {}
# 🌉 Bridging
Bridging in Blitz is limited to the bridging of Relics into the game. This is to prevent players from gaining advantage
through the import of materials previously extracted from Eternum seasons, while still encouraging the use of
consumables for engaging and competitive gameplay. There is no bridging out of Blitz sessions, once relics are bridged
in they cannot be taken out of the session.
## Bridging Relics
*Work in Progress... Initial versions of Blitz will only feature Relics found within
[Relic Crates](/blitz/worldmap-movement/worldmap#relic-crates). More information on tokenized Relics will be made
available soon*
---
file: docs/pages/blitz/materials/production.mdx
meta: {}
import {
BlitzDonkeyProduction,
BlitzStandardTroopProduction,
BlitzSimpleTroopProduction,
BlitzSimpleResourceProduction,
BlitzStandardResourceProduction,
BlitzLaborProduction,
} from "@/components/ResourceProduction";
# ⚒️ Production
## Standard vs. Simple
As is the case for building construction, Lords can choose between standard and simple production systems when deciding
how they wish to produce their materials. This can be done by selecting the appropriate panel when making an order in
the production screen.
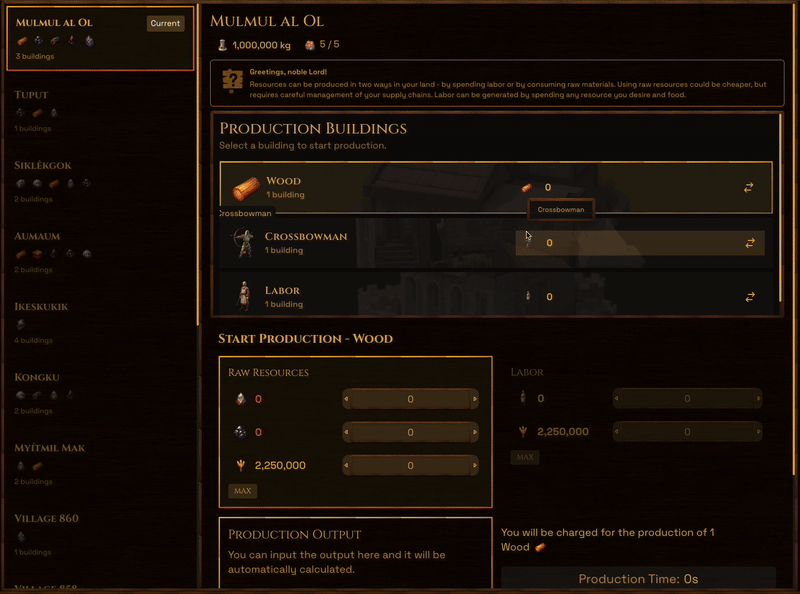
*Note: Lords must commit the full material requirements to the production order upfront. The output is then produced at
a given rate per second.*
### Standard Mode
The classic production system—every resource and troop production order requires food and a combination of other
resources as inputs. As with buildings, standard mode is the more complex but efficient way to produce materials.
### Simple Mode
Simple mode reduces the requirements for a production order to food and labor. This is, once again, less efficient in
both time and resources—but it allows Lords to produce resources on their Realm even if they haven't yet aquired the
input resources required for standard mode production.
## Materials Production
### Food
Wheat is the only food source in Blitz and can be produced without inputs. Once a Farm has been constructed, it can
produce food indefinitely. Each Farm produces 5 Wheat per second.
### Resources
The 9 resources found in Blitz are the backbone of the economy. Producing one resource requires the input of two other
resources (standard mode), or labor (simple mode). Resources are also the main components in building construction and
troop production - so mastery of these assets is key to success and security.
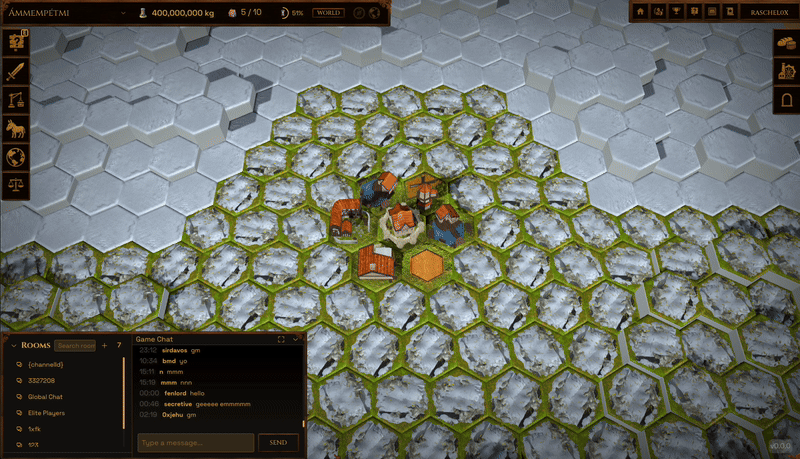
### Labor
Labor is the primary input for construction and production in simple mode, but is also required in standard mode for
building construction and Realm upgrades. It is produced by permanently burning resources in the Keep. This creates a
steady stream of Labor at a fixed rate. Each of the 9 resources can be burned to produce Labor at varying efficiency,
dependent on rarity. The amount of resources burned per second to produce Labor at the fixed rate is as follows:
### Troops
Troops are essential for exploration, defending your lands and conquering your enemies. Every Lord will be required to
produce troops to ensure they remain a relevant force throughout the game. Three troop tiers are available, each
requiring a combination of food, rare resources, and troops of the previous tier as input for production.
*Note: Only T1 troops can be produced in both standard and simple mode. T2 and T3 troops can only be produced in
standard mode.*
### Donkeys
The humble steeds that move all materials around the world must themselves be produced in a market building. Donkeys are
one-time-use and consumed after a single journey, so Lords will need to produce a steady supply of donkeys to ensure
that they can sustain their supply chains and logistics. Once the market has been constructed, donkeys only require a
steady stream of food to produce.
---
file: docs/pages/blitz/materials/relics.mdx
meta: {}
# 🏺 Relics
Relics are powerful consumables that can be discovered in Blitz from
[Relic Crates](/blitz/worldmap-movement/worldmap#relic-crates), or rewarded for playing Blitz games through Relic
Chests. Depending on the nature of a Relic, it can be consumed by an army or a Realm in exchange for a time-limited
bonus, such as a damage or production boost. When utilized at the right time, these items can provide a strategic edge
over your opponents.
*Note: Relics are still under development. Initial versions of Blitz will limit their scope to within the game instance.
However, it is expected that Relics will have a much broader presence and impact in the Realms ecosystem. Keep an eye
out for announcements in the Realms Discord relating to Relics. This page will be updated as more information is made
available to the community.*
---
file: docs/pages/blitz/materials/resources.mdx
meta: {}
import RarityResourceTable from "@/components/RarityResourceTable";
import ResourceIcon from "@/components/ResourceIcon";
import { ResourcesIds } from "@bibliothecadao/types";
# 🧱 Materials
## Materials Overview
Materials encompass all transportable assets in Blitz, including:
* **Resources**: 9 distinct resource types that can be produced on Realms.
* **Food**: Essential wheat that fuels production and troop movement.
* **Troops**: Military units (Knights, Crossbowmen, Paladins) that can be assigned to armies.
* **Donkeys**: One-time-use transport units required for moving materials across the world.
* **Labor**: A special material that enables construction and production.
* **Essence**: A new strategic resource found through exploration and required for building, production, and upgrades.
While all materials can be transported within the game, **no materials can be bridged out** of Blitz. Players may only
bridge Relics into the game.
## Material Categories
### Resources
Resources are the foundation of the economy. The 9 resources play vital roles in Blitz, from basic production to
advanced military operations.
### Food
Food is the cornerstone of a Realm's economy and is required in all production chains. Only wheat is available in Blitz
and it can be produced without inputs.
### Troops
Troops are required to defend your holdings and strike out at your foes. When produced, they can be transported between
your Realms by donkey like any other material, but to be used they must be deployed to an army. Once deployed, they
cannot be converted back into material form.
### Donkeys
Donkeys are essential for moving materials between your Realms. They only require a market and wheat to produce, but are
consumed after a single journey.
### Labor
Labor is a key component in the construction of all buildings and required for 'simple mode' production. It is produced
by burning resources, with rarer resources yielding more labor.
### Essence
Essence is a new material that can only be found by exploring the map and interacting with World Structures. It is
required in all Realm upgrades, as well as for some building construction and production of high-tier troops.
---
file: docs/pages/blitz/materials/storage.mdx
meta: {}
import { WeightTable } from "@/components/WeightTable";
import { formatNumberWithCommas } from "@/utils/formatting";
import { divideByPrecision } from "@/utils/resources";
import { CapacityConfig, ResourcesIds } from "@bibliothecadao/types";
import { ETERNUM_CONFIG } from "@/utils/config";
import { BlitzWeightTable } from "@/components/WeightTable";
import { StorageTable } from "@/components/StorageTable";
# 📦 Storage System
Your available storage limits the amount of materials your Realm can hold. Each Realm begins with a base capacity of
**20,000 kg**. You'll need to construct storehouses to expand your storage capabilities.
## Material Weights
Materials have different weights that affect storage:
## Storehouses
Each storehouse adds 20,000 kg of storage capacity. Lords must be careful not to exceed the storage capacity of their
Realms, as any produced materials that cannot be stored will be permanently lost.
## World Structure Storage
All world structures have set storage capacities **(that cannot be expanded through the use of storehouses)**. These
capacities are as follows:
---
file: docs/pages/blitz/materials/transfers-and-trade.mdx
meta: {}
import { importantNote } from "@/components/styles";
# 📜 Transfers
Blitz has no trade system. Blitz focuses on individual competitive performance without economic trading mechanics. As
such, there are no Banks, no P2P transfers, and no AMM or Orderbook through which Lords may trade.
## Transferring Materials
Throughout the game, Lords will face the need to transfer materials from place to place around the world map, whether it
be to their own Realms, or other structures like Hyperstructures, Essence Rifts and Camps. Lords have two primary
options for initiating transfers:
* **Detailed Transfer**: Players can use the comprehensive transfer menu accessible from the Trade tab. This allows
transfers of multiple types of materials simultaneously, to any structure on the map.
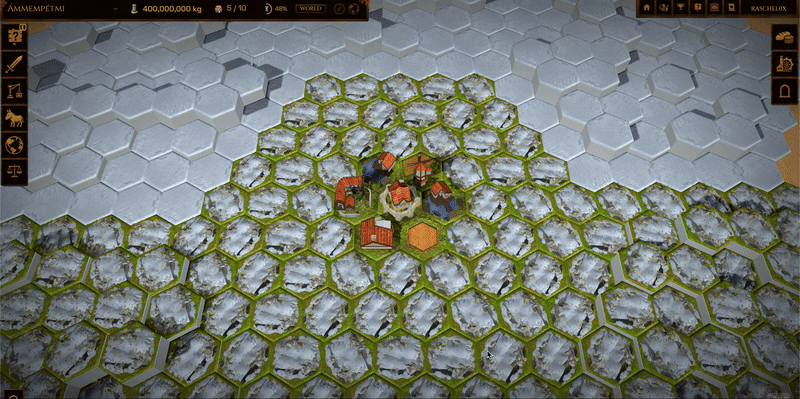
* **Quick Transfer**: Alternatively, Lords can swiftly transfer resources between their own structures using the
resources sidebar, ideal for managing internal logistics and maintaining supply chains.
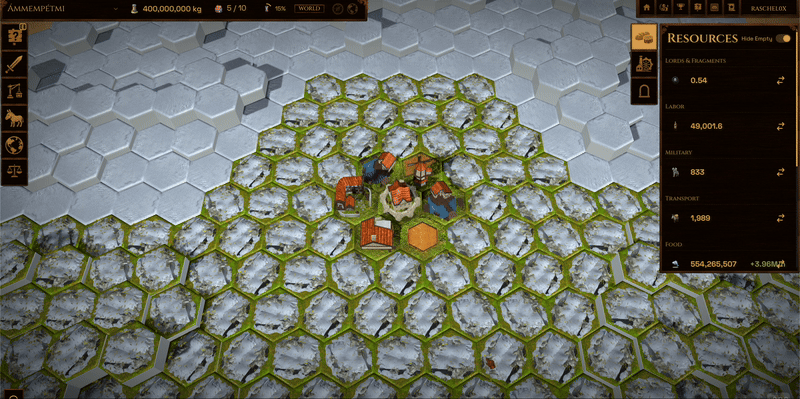
## The Donkey Network
When a transfer is initiated, donkeys travel through the invisible and immutable donkey network. This is the key
infrastructure upon which all material transport takes place. One donkey is capable of carrying up to **50 kg** of
materials, so the number of donkeys required for a transfer depends on the total weight of the materials being moved.
Once a transfer is initiated, it cannot be canceled, and the journey will take some time depending on the distance to
the destination structure. Upon arrival, transferred materials and donkeys must be manually claimed via the 'Resource
Arrivals' tab before they become available for use.
### Blitz Restrictions
While troops can be transferred between Realms as normal, donkeys cannot be used to transport troops between Realms and
Hyperstructures, Essence Rifts or Camps. This is to reduce the defensive advantage that a player can leverage by being
able to move troops quickly into guard armies on these structures once claimed. All players must therefore deploy armies
from Realms and move them across the map to contest these critical points.
---
file: docs/pages/blitz/military/armies.mdx
meta: {}
import {
RealmDefenseSlotsTable,
WorldStructureDefenseSlotsTable,
BlitzWorldStructureDefenseSlotsTable,
} from "@/components/DefendingArmies";
import BlitzTroopsLimitTable from "@/components/BlitzTroopsLimitTable";
import { ETERNUM_CONFIG } from "@/utils/config";
import { formatNumberWithCommas } from "@/utils/formatting";
# ⚔️ Armies
Individual armies consist of a single troop type and tier. Each hex can host only one army, so Lords must carefully
manage their positioning and engagement opportunities.
Each Realm has a limited number of guard and field armies. The number of armies can be increased by upgrading the Realm
level as explained in the [Realms](/blitz/realms/realm) section. Each military building constructed also increases total
field armies by 1 (up to a maximum of **+{ETERNUM_CONFIG().troop.limit.explorerMaxPartyCount-4}**). Lords can deploy
armies from the Military tab in the Local view. The maximum size of an army is
**{formatNumberWithCommas(ETERNUM_CONFIG().troop.limit.explorerAndGuardMaxTroopCount)}**.
## Field Armies
Field armies are used to project force on the world map, explore unknown territories, claim hyperstructures, defeat
bandit forces, and engage in combat with other players. These armies are deployed onto one of the six hexes surrounding
their parent Realm on the world map. If all hexes adjacent to the Realm are occupied, a field army cannot be deployed.
Once deployed, these armies occupy a hex and traverse the world map based on the conditions outlined in the
[World Map & Movement](/blitz/worldmap-movement/movement) section.
Lords may direct their field armies to attack another army or structure on any adjacent hex by selecting the army with
left-click, then right-clicking the target hex. When attacking a structure, the field army will engage in combat against
any guard armies present.
Flow to create field army in the client
## Guard Armies
Guard armies do not appear on the world map; instead, they occupy defense slots visible in the Military tab of the Local
view. When in the World view, Lords can observe the defensive capabilities of any structure by selecting a hex and
opening up the Details tab.
Unlike Realms, World Structures have a static number of defense slots:
Flow to create guard in the client
When attacked, guard armies are targeted sequentially starting from the outermost occupied slot. If a guard army is
destroyed, the slot that it occupied becomes unavailable for reinforcement for **10 minutes**. Once all guard armies are
defeated, the structure becomes vulnerable to being claimed by an adjacent field army. Lords can also direct their guard
armies to launch attacks against enemy field armies on adjacent hexes by selecting their structure on the world map,
then right-clicking the target hex.
Attack field army with structure
---
file: docs/pages/blitz/military/damage.mdx
meta: {}
# 💥 Damage
import { DamageCalculation } from "@/components/DamageCalculation";
## Damage Overview
Damage is defined as the number of casualties dealt by one army against the other in an instance of combat. When combat
is initiated (i.e. one army attacks another), damage for both the attacking and defending army is calculated based on
the number of troops, troop tier, stamina, and biome bonuses. Damage is then simultaneously inflicted by both sides.
Battles can last multiple rounds (requiring multiple combat instances) depending on the relative strength and number of
troops involved. Armies with higher-tier troops deal significantly more damage. However, careful management of troop
numbers, stamina, and biome positioning can enable lower-tier armies to overcome stronger opponents.
---
file: docs/pages/blitz/military/raiding.mdx
meta: {}
# 💰 Raiding
DRAFTING NOTE: Suggest we just remove raiding from Blitz, there's no point in it.
---
file: docs/pages/blitz/military/stamina-and-biomes.mdx
meta: {}
import { BiomeCombat } from "@/components/BiomeCombat";
# ⚡ Stamina & Biome Effects
## Stamina
Stamina is a vital resource governing the mobility and effectiveness of armies. Each deployed army has a maximum stamina
value determined by its troop type and tier as explained above. Field armies expend stamina when moving, exploring
unknown hexes, or engaging in combat against other armies and structures. Guard armies only expend stamina when
attacking armies in hexes adjacent to their structure. During combat, stamina impacts army combat effectiveness:
### Attacking Army
The attacking army is the army initiating an instance of combat. Attacks require a minimum of **30 stamina**, so armies
with less than 30 stamina cannot initiate an attack. Each additional stamina point above this threshold grants a **1%**
damage bonus, up to a maximum of **+30%** damage at **60 stamina**. Additional stamina spend is not optional, so
attacking armies will use all available stamina up to a maximum of **60** for any one attack. If the attacking army
destroys the defending army, they gain **+30 stamina**.
### Defending Army
The defending army is the army being attacked in an instance of combat. Defending armies rely on having stamina
available to maintain full combat effectiveness. When attacked, defending armies incur a **1%** damage reduction for
every stamina point below **30**. For example, if an army is attacked while they have **10 stamina**, they would deal
**-20%** damage to the attacking army. The maximum penalty is **-30%** damage if stamina is completely depleted.
Successfully defending an attack does not deplete stamina. Lords should manage their armies' stamina carefully, ensuring
they retain enough reserves to remain effective against unexpected attacks.
## Biomes (Combat Effects)
The combat effectiveness of different troop types is impacted by the biome on which the defending army is located when
engaging in battle. Armies can gain either a combat advantage (**+30%** damage), disadvantage (**-30%** damage), or no
effect, depending on their affinity with a particular biome. To summarise these combat modifiers for each troop type:
⚔️️ ️️️**Knights** excel in forests, leveraging cover and mobility, but suffer reduced effectiveness in deserts,
beaches, and snow-covered regions where their heavy armour and weapons can make it difficult to move on the soft ground
cover. Knights are equally advantaged, disadvantaged or not affected across all biome types.
🏹 **Crossbowmen** benefit from open seas and unstable terrains (such as beaches and snow), where they can remain static
and make the most of their ranged capabilities. However, they will be disadvantaged in flat, open terrain with no cover
or opportunity for concealment. Crossbowmen are the least advantaged, but also the least disadvantaged by biome
modifiers of the three troop types.
️**Paladins** dominate open, flat terrain where mounted units thrive, but are hindered in dense forests and on open
water where their mounted capabilities are negated. They are the most advantaged, but also the most disadvantaged troop
type. In combination with the biome effects on stamina use, Paladins are less versatile than Knights or Crossbowmen, but
are devastatingly effective in the right situations.
**Note: The scorched biome applies +30% damage to all troop types, representing the additional casualties sustained from
battling in an inhospitable environment.**
---
file: docs/pages/blitz/military/troop-tiers.mdx
meta: {}
import { TroopTiers, TroopTierDamageStats } from "@/components/TroopTiers";
import { MaxStaminaTable } from "@/components/TroopMovementTable";
# 🛡️ Troop Tiers
Troops in Eternum come in three distinct tiers: T1, T2, and T3. Tier 1 (T1) represents basic troops, while T2 and T3
troops are more advanced, offering significantly increased combat strength and stamina.
Higher-tier troops are produced by constructing specialized buildings that require rare resources and lower-tier troops
as inputs:
* Two T1 troops combine to produce one T2 troop
* Two T2 troops combine to produce one T3 troop
Each troop tier provides escalating advantages in terms of damage output and stamina.
---
file: docs/pages/blitz/realms/buildings.mdx
meta: {}
import BuildingCard from "../../../components/BuildingCard";
import { formatNumberWithCommas } from "@/utils/formatting";
import { CapacityConfig, resources, ResourcesIds } from "@bibliothecadao/types";
import { ETERNUM_CONFIG } from "@/utils/config";
import { BlitzBuildingCard } from "@/components/BlitzBuildingCard";
# 🪓 Buildings
## Standard vs. Simple
Blitz offers two building systems. Lords can switch between standard and simple building modes at any time by clicking
the toggle at the top of the construction tab.
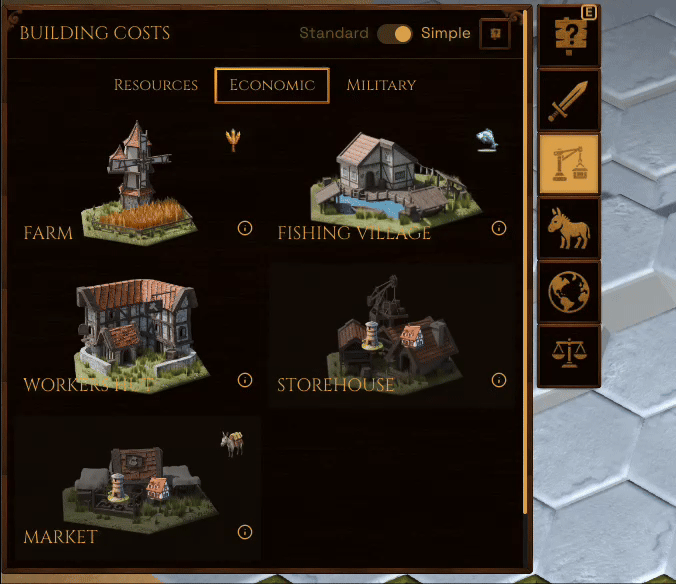
*Note: Some buildings only require labor to construct. Farms and Wood Mills have the same construction cost in both
standard and simple building modes.*
### Standard Mode
This is the classic building experience. Construction requires a combination of labor and a particular set of resources
as building materials. Standard mode is more complex and involves a greater number of materials in storage, however it
is far more efficient overall.
### Simple Mode
This mode allows players to utilize Labor as the singular input for construction. Simple mode greatly improves
accessibility and simplifies gameplay overall, but is much less efficient in both time and materials.
## Placement & Population
### Placement
The construction tab can be accessed in the Local view. Buildings can be constructed on any buildable hex of a Realm,
provided the owner has the requisite materials and population capacity. Buildable hexes are defined by the upgrade level
(Settlement, City, Kingdom, or Empire) and are visible as hexes with patches of bare, prepared earth.
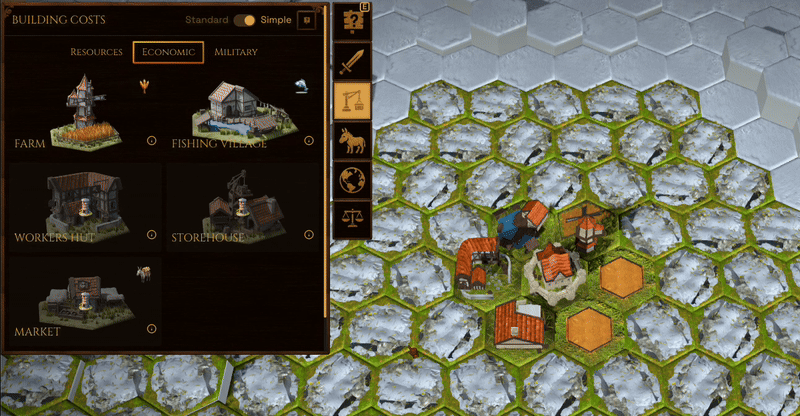
### Population
**Population** is the total population used to construct the current buildings on a Realm, while **population capacity**
is the maximum available population with which to construct buildings. Worker Huts can be built to increase population
capacity, while all other buildings require population capacity to be available in order to construct. Lords must
balance their construction to ensure there is enough population capacity available to construct their desired production
buildings.
## Building Types
### The Keep
All Realms start the game with a structure in the center of the buildable hexes, known as the Keep. This structure
represents the central point of governance of the Realm, and facilitates key functions such as Realm upgrades and labor
production. This building cannot be destroyed and provides a baseline population capacity of 5.
### Resource Buildings
There is a specific resource building for each of the 9 resources in Blitz, so Lords will require separate resource
buildings if they wish to produce more than one type of resource. These buildings only require food to construct and
have the same requirements in simple and standard mode.
### Economic Buildings
These buildings define the utility of a Realm or Village, including food production, donkey production, population
capacity, and storage capacity. All of these buildings can be constructed in either standard or simple mode, but have
varying costs and population requirements:
### Military Buildings
Your military buildings are essential for training up armies to defend your territories and project your might. There
are three 'tiers' of each of the three troop types, but a different building is required to produce each troop tier. As
such, there are a total of 9 military buildings in the game.
Note: Only T1 troop buildings can be constructed in both standard and simple mode. T2 and T3 military buildings are only
available for construction in standard mode.
### Same Building Cost Increase
Building multiple buildings of the same type will cost progressively more each time. The cost increase is calculated
using the following formula:
```
Cost = Base Cost + (Base Cost × 0.1 × (N-1)²)
```
Where:
* **Base Cost** is the initial cost of the building (from the tables above)
* **N** is the number of the same buildings already constructed
For example, if you build a second building of the same type (N=2), the cost will be:
```
Cost = Base Cost + (Base Cost × 0.1 × (2-1)²)
= Base Cost + (Base Cost × 0.1 × 1)
= Base Cost × 1.1
```
*Note: Buildings need to be exactly the same type for this rule to apply. For example, building a Coal Mine after a Wood
Mill (both being resource buildings) would not be subject to this cost increase.*
---
file: docs/pages/blitz/realms/realm.mdx
meta: {}
import { StartingMilitary } from "@/components/StartingMilitary";
import { BlitzRealmDefenseSlotsTable } from "@/components/DefendingArmies";
import { BuildableHexes } from "@/components/BuildableHexes";
import { RealmLeveling } from "@/components/RealmLeveling";
import ResourceIcon from "@/components/ResourceIcon";
import { importantNote, table, formatAmount, section } from "@/components/styles";
import { BlitzRealmLeveling } from "@/components/BlitzRealmLeveling";
# 🏰 Realms
In Blitz, each Lord starts the game commanding three Realms, evenly spaced in a triangular formation. These Realms are
functionally identical and are not related to the 8,000 Realm NFTs that are the basis of Eternum. They can produce any
of the nine resources available in Blitz and are equally capable in all aspects of the game, limited only by the skill
and strategy of their Lord.
## Registration
All Lords willing to participate in a Blitz game are required to register prior to the start of the Settling Phase. The
'Registration Phase' is different for each bracket, but in all cases it is used to predetermine the number of players
and establish the size of the game world. Each wallet address can only be registered once per Blitz game. More
information on Blitz registration requirements will be made available prior to launch.
## Settling
Realms are settled during the 30-minute 'Settling Phase' prior to the start of the game. During this phase, registered
Lords must confirm their participation in the game, after which their three Realms will be placed in a randomly assigned
position. Lords who do not confirm participation within this timeframe will forfeit their entry into the session,
leaving a potential spawn position empty throughout the game.
### Settling Algorithm
The number of registered Lords for a session determines the size of the map and the number of Hyperstructures available
for Lords to compete over. A single Hyperstructure marks the center of the map, around which other concentric
Hyperstructure 'rings' are spawned every 18 hexes until all players are accommodated. The details of Hyperstructure
spawns are explained in greater detail in the [World Map](/blitz/worldmap-movement/worldmap) section.
Once the size of the map and the number of Hyperstructures are determined, player positions are determined within the
bounds of the Hyperstructure rings. While the order in which players are placed is randomised, the positioning is
sequential and follows a rotational pattern to distribute Lords as evenly as possible and attempt to ensure equal
opportunity in accessing the Hyperstructure objectives. The map structure and placement sequence for an example of 24
players is visualized below:
⚠️ IMPORTANT NOTE
There is no immunity period in Blitz. All Realms are able to be attacked and claimed from the moment the game
begins.
## Realm Details
### Starting Resources
Starting Resources per Realm |
Quantity |
Labor
|
5,000 |
Donkeys
|
200 |
T1 Troops
|
3,000 |
*Note: The type of T1 troop depends on the biome that the realm is spawned on. Starting troops will always have a combat
advantage when defending their home.*
### Realm Progression
Realms can grow from a Settlement into a City, then to a Kingdom, and finally an Empire. Each upgrade level unlocks
additional buildable hexes and defensive army slots, allowing for the expansion of your economy and strengthening of
your defenses. Each progression requires specific materials, outlined in the table below:
---
file: docs/pages/blitz/worldmap-movement/movement.mdx
meta: {}
import { BlitzTroopMovementTable } from "@/components/TroopMovementTable";
import { BiomeStamina } from "@/components/BiomeStamina";
import { ExplorationRewards } from "@/components/ExplorationRewards";
# 🧭 Movement & Exploration
## Stamina
Stamina is expended whenever an army moves between hexes, explores previously unrevealed areas, or launches an attack
against enemy forces. Each of these actions has a specific stamina cost, making strategic planning essential to
maximizing an army's effectiveness. Armies regenerate stamina at a consistent rate of +60 stamina per Eternum Day. Each
troop type possesses different maximum stamina capacities, outlined in the table below:
Lords should remain cautious about fully depleting the stamina of their armies, as forces with low stamina may find
themselves severely disadvantaged if attacked by enemy forces.
## Movement
Armies can move freely into any adjacent, unoccupied hex. A hex is considered occupied if it contains a Realm, another
army, or a world structure such as a Hyperstructure. Armies may also move to more distant hexes, provided there is a
continuous path of adjacent, unoccupied hexes leading to the destination, and the army has sufficient stamina available
for the entire journey.
Moving armies in the client
The amount of stamina consumed when moving into a hex depends on both the biome of that hex and the type of troops
within the army. The table below details biome-specific stamina costs for each troop type:
## Exploring
Exploring your surroundings is a necessary part of Blitz as it allows Lords to reveal paths to objectives, find valuable
Essence or other bonuses, and uncover beneficial world structures.
When an army is positioned adjacent to an unexplored hex, Lords may choose to send that army forth to uncover what lies
hidden. Exploring an unknown hex always costs 30 stamina and will reveal that hex to all Lords, permanently expanding
the known boundaries of the world. If the newly explored hex contains a World Structure, the exploring army will
automatically be pushed back to the hex from which they started without any additional stamina penalty.
During exploration, armies gather any valuables they encounter, receiving the goods directly into their inventory. The
reward chances for any explored hex are as follows:
If an army doesn't have sufficient carrying capacity to store the discovered materials, the materials will be
permanently lost (burned) but the army will be able to continue exploring provided they have enough stamina. Armies can
return to a structure to offload the materials, or drop them to free up space. Note that any materials discarded in the
field will also be burned.
Explore in the client
## Troop Transfers
Lords may transfer troops from one army to another, provided that they are on adjacent hexes, the same troop type, and
the same tier. To transfer troops from one army to another, or to a structure, the player first needs to select its army
on the worldmap using left-click, then right-click on the target and select the Transfer -> Transfer Troops tab.
Transferring troops from one army to another with lower stamina makes no change to the stamina of either army.
Transferring from an army with lower stamina to an army with higher stamina will reduce the higher-stamina army to equal
that of the lower stamina army.
Transfer troops in the client
---
file: docs/pages/blitz/worldmap-movement/worldmap.mdx
meta: {}
import { HyperstructureRings } from "@/components/HyperstructureRings";
import { importantNote } from "@/components/styles";
# 🗺️ The World Map
## An Unexplored World
At the beginning of each Blitz game, the world lies largely unexplored. The only exceptions are the settled Realms and
the array of Hyperstructures which can be seen in the World view, arranged in expanding rings around the center of the
map. The six hexes adjacent to these structures are also revealed, but beyond these instances, the world remains cloaked
by the mist, waiting for ambitious Lords to send forth their armies to explore. Once a hex is explored, the state of the
hex (i.e. the biome and whether it contains a world structure) is permanently revealed to all Lords.
## Map Layout
### Hyperstructure Positioning
The number of registered Lords for a game of Blitz determines the size of the map and the number of Hyperstructures
available for Lords to compete over. A single Hyperstructure marks the center of the map, around which other concentric
Hyperstructure 'rings' are spawned every 18 hexes until all players are accommodated.
### Realm Positioning
Once the size of the map and the number of Hyperstructures are determined, player positions are determined within the
bounds of the Hyperstructure rings. While the order in which players are placed is randomised, the positioning is
sequential and follows a rotational pattern to distribute Lords as evenly as possible and attempt to ensure equal
opportunity in accessing the Hyperstructure objectives.
The Realms of each participating Lord are positioned six hexes apart in a triangle formation. Each Realm is also located
within eight hexes of a Hyperstructure, and eight hexes from the nearest Realm belonging to another Lord (assuming all
available player positions are filled). More details of the positioning system are available in the
[Realms](/blitz/realms/realm) section.
⚠️ IMPORTANT NOTE
There is no immunity period in Blitz. All Realms are able to be attacked and claimed from the moment the game
begins.
## Relic Crates
An unknown force occasionally reveals treasures from the past in random locations on the world map. As players explore
hexes, there is a chance that a Relic Crate will be revealed on a different nearby tile, visible to all players. These
crates can contain powerful boosts that can accelerate the production of your Realms, improve the stamina regeneration
of your armies, or even give them combat damage boosts to give you an edge over your foes. The first army to reach and
activate the crate will be receive the contents directly into their inventory, so be sure to secure them before your
enemies do!
---
file: docs/pages/eternum/military/armies.mdx
meta: {}
import { RealmDefenseSlotsTable, WorldStructureDefenseSlotsTable } from "@/components/DefendingArmies";
import { ETERNUM_CONFIG } from "@/utils/config";
import { formatNumberWithCommas } from "@/utils/formatting";
# ⚔️ Armies
In Season 1, individual armies consist of a single troop type and tier—streamlining combat strategy and simplifying
interactions on the world map. Each hex can host only one army, so Lords must carefully manage their positioning and
engagement opportunities.
Each Realm and Village has a limited number of guard and field armies The number of armies can be increased by upgrading
the Realm / Village level as explained in the [Realms](/eternum/realm-and-villages/realm) section. Each military
building constructed on a Realm or Village also increases total field armies by 1 (up to a maximum of
**+{ETERNUM_CONFIG().troop.limit.explorerMaxPartyCount-4}**). Lords can deploy armies from the Military tab in the Local
view. The maximum size of an army is
**{formatNumberWithCommas(ETERNUM_CONFIG().troop.limit.explorerAndGuardMaxTroopCount)}**.
### Field Armies
Field armies are used to project force on the world map, explore unknown territories, patrol borders, defend key areas,
or march against your enemies. These armies are deployed onto one of the six hexes surrounding their parent Realm on the
world map. If all hexes adjacent to the Realm are occupied, a field army cannot be deployed. Once deployed, these armies
occupy a hex and traverse the world map based on the conditions outlined in the World Map & Movement section.
Lords may direct their field armies to attack another army or structure on any adjacent hex by selecting the army with
left-click, then right-clicking the target hex. When attacking a structure, the field army will engage in combat against
any guard armies present.
Flow to create field army in the client
### Guard Armies
Guard armies do not appear on the world map; instead, they occupy defense slots visible in the Military tab of the Local
view. When in the World view, Lords can observe the defensive capabilities of any structure by selecting a hex and
opening up the Details tab.
Unlike Realms and Villages, world structures have a static number of defence slots:
Flow to create guard in the client
When attacked, guard armies are targeted sequentially starting from the outermost occupied slot. If a guard army is
destroyed, the slot that it occupied becomes unavailable for reinforcement for
**{Math.floor(ETERNUM_CONFIG().troop.limit.guardResurrectionDelay / 3600)} hours**. Once all guard armies are defeated,
the structure becomes vulnerable to being claimed by an adjacent field army (with the exception of Villages, which
cannot be claimed). Lords can also direct their guard armies to launch attacks against adjacent hexes by selecting their
structure on the worldmap, then right-clicking the target hex.
Attack field army with structure
---
file: docs/pages/eternum/military/damage.mdx
meta: {}
# 💥 Damage
import { DamageCalculation } from "@/components/DamageCalculation";
## Damage Overview
Damage is defined as the number of casualties dealt by one army against the other in an instance of combat. When combat
is initiated (i.e. one army attacks another), damage for both the attacking and defending army is calculated based on
the number of troops, troop tier, stamina, and biome bonuses. Damage is then simultaneously inflicted by both sides.
Battles can last multiple rounds (requiring multiple combat instances) depending on the relative strength and number of
troops involved. Armies with higher-tier troops deal significantly more damage. However, careful management of troop
numbers, stamina, and biome positioning can enable lower-tier armies to overcome stronger opponents.
---
file: docs/pages/eternum/military/raiding.mdx
meta: {}
import { ETERNUM_CONFIG } from "@/utils/config";
import { resource } from "@/components/styles";
import { RaidableResources } from "@/components/RaidableResources";
import { importantNote } from "@/components/styles";
# 💰 Raiding
Raiding allows field armies to attempt theft of valuable resources directly from an adjacent target structure, without
first having to battle through enemy guard armies. Lords can direct their armies to complete this action by selecting
the 'raid' action when attacking an enemy structure.
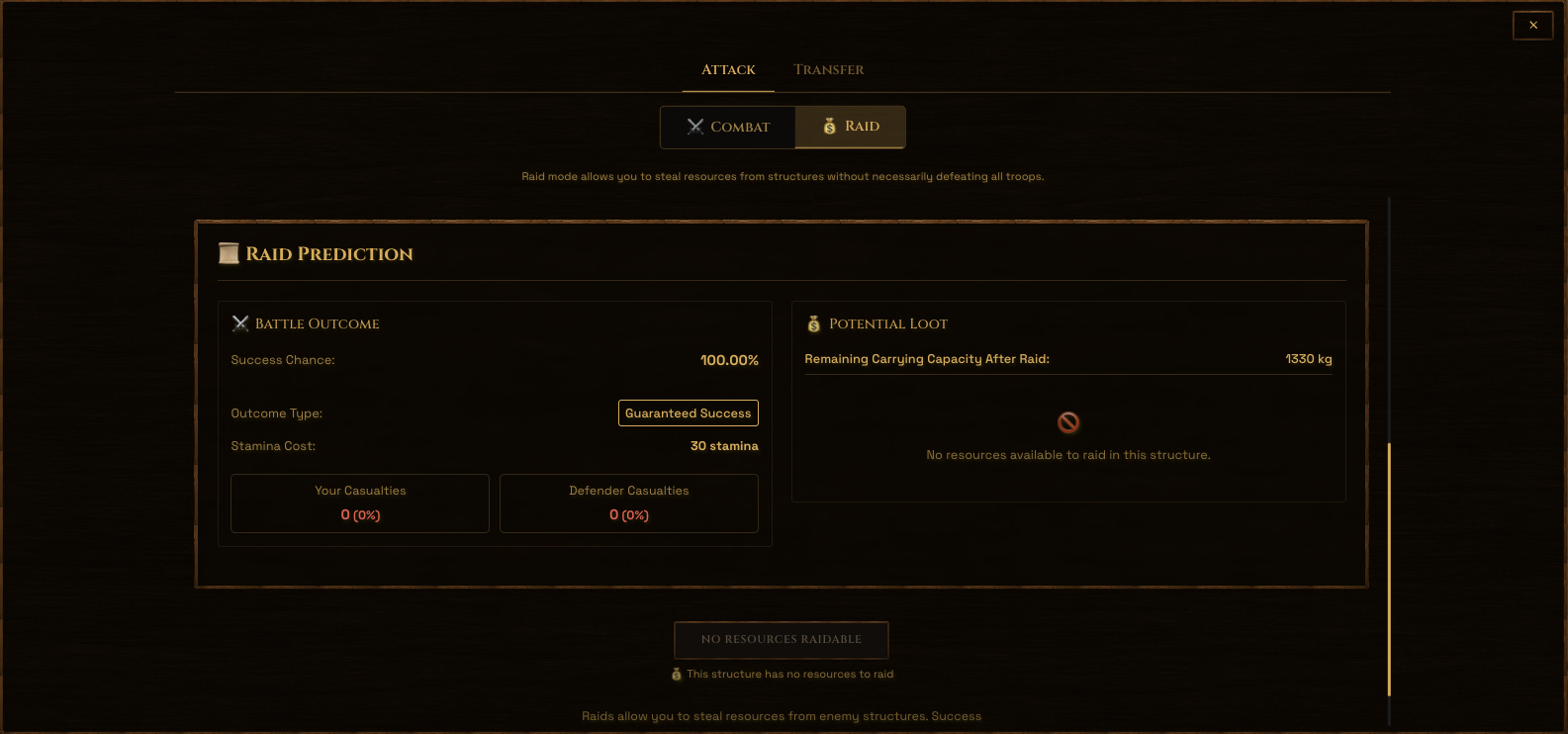
## Raid Requirements
Before attempting a raid, your army must meet the following requirements:
* Have at least **{ETERNUM_CONFIG().troop.stamina.staminaAttackReq}** stamina
* Be adjacent to the target structure
* Structure must contain raidable resources
## Raidable Materials
Raidable materials are stolen based on rarity, with the most valuable taken first. Rarer materials are prioritized
during raids:
⚠️ IMPORTANT NOTE
No materials are safe from pillagers, $LORDS tokens can be raided as well
## Raid Success
The success chance of a raid is determined by the following conditions:
| Condition | Raid Success Chance |
| -------------------------------------------------------------------------- | --------------------------------------------- |
| Raiding army's total damage \< **50%** of combined guard armies' damage | **0%** |
| Raiding army's total damage > **200%** of combined guard armies' damage | **100%** |
| Raiding army's damage between **50%** and **200%** of guard armies' damage | Scales proportionally from **0%** to **100%** |
| No guard armies present | **100%** (automatic success) |
Upon a successful raid, resources are transferred directly to the raiding army's inventory up to its carrying capacity.
It is only possible to steal food, resources, and ancient fragments from a raid, tokens such as $LORDS and $STRK can
only be stolen from another player by claiming a Realm into which tokens have been bridged or transferred.
## Damage in Raids
At the conclusion of a raid, regardless of whether it was successful or not, damage is calculated for both the raiding
army and the guard armies. This damage is significantly reduced by a raid damage modifier, simulating the concept of a
pillaging and looting event as opposed to a full-scale battle. The raid damage modifier reduces damage to
**{ETERNUM_CONFIG().troop.damage.damageRaidPercentNum/100}%** of that which would be applied in an attack action.
Damage from a raiding action is calculated according to the following rules:
* The raiding army troop total is split evenly across each guard army.
* A standard combat damage calculation is then applied to each combat instance.
* Each damage number is multiplied by the raid damage modifier before casualties are applied.
### Example:
Consider **1000** raiders attacking a structure with two occupied guard slots:
* Slot 1: **400** troops
* Slot 2: **600** troops
The raiding army is split into two groups of **500** troops, each attacking one guard slot. Assume that the damage
calculations result in the following:
* Raiding army deals **180** damage to Slot 1 and **150** damage to Slot 2 = **330** total damage
* Guard armies deal **120** damage from Slot 1 and **200** damage from Slot 2 = **320** total damage.
Applying the raid damage modifier, actual losses become:
* Guards: Slot 1 loses **36** troops (**{ETERNUM_CONFIG().troop.damage.damageRaidPercentNum/100}%** of 180), Slot 2
loses **30** troops (**{ETERNUM_CONFIG().troop.damage.damageRaidPercentNum/100}%** of 150).
* Raiders: Lose **64** troops total (**{ETERNUM_CONFIG().troop.damage.damageRaidPercentNum/100}%** of 320).
## Raid Outcomes
After a raid attempt, one of two outcomes will occur:
1. **Successful Raid**
* Resources are transferred to your army's inventory
* Resources are limited by your army's carrying capacity
* Both armies suffer reduced casualties (**{ETERNUM_CONFIG().troop.damage.damageRaidPercentNum/100}%** of normal
combat damage)
* Your army's stamina is reduced by **{ETERNUM_CONFIG().troop.stamina.staminaAttackReq}**
2. **Failed Raid**
* No resources are stolen
* Both armies still suffer reduced casualties (**{ETERNUM_CONFIG().troop.damage.damageRaidPercentNum/100}%** of
normal combat damage)
* Your army's stamina is still reduced by **{ETERNUM_CONFIG().troop.stamina.staminaAttackReq}**
The outcome is determined by comparing your army's total damage output against the combined damage output of all guard
armies present. If no guard armies are present, the raid automatically succeeds.
---
file: docs/pages/eternum/military/stamina-and-biomes.mdx
meta: {}
import { BiomeCombat } from "@/components/BiomeCombat";
# ⚡ Stamina & Biome Effects
## Stamina
Stamina is a vital resource governing the mobility and effectiveness of armies. Each deployed army possesses a maximum
stamina value determined by its troop type and tier as explained above. Field armies expend stamina when moving,
exploring unknown hexes, or engaging in combat against other armies and structures. Guard armies only expend stamina
when attacking armies in hexes adjacent to their structure. During combat, stamina impacts army combat effectiveness:
### Attacking Army (the army initiating combat)
Attacks require a minimum of **30 stamina**, so armies with less than 30 stamina cannot initiate an attack. Each
additional stamina point above this threshold grants a **1%** damage bonus, up to a maximum of **+30%** damage at **60
stamina**. Additional stamina spend is not optional, so attacking armies will use all available stamina up to a maximum
of **60** for any one attack. If the attacking army destroys the defending army, they gain **+30 stamina**.
### Defending Army (the army being attacked)
Defending armies rely on having stamina available to maintain full combat effectiveness. When attacked, defending armies
incur a **1%** damage reduction for every stamina point below **30**. For example, if an army is attacked while they
have **10 stamina**, they would deal **-20%** damage to the attacking army. The maximum penalty is **-30%** damage if
stamina is completely depleted. Successfully defending an attack does not deplete stamina. Lords should manage their
armies' stamina carefully, ensuring they retain enough reserves to remain effective against unexpected attacks.
## Biomes (Combat Effects)
The combat effectiveness of different troop types is impacted by the biome on which the defending army is located when
engaging in battle. Armies can gain either a combat advantage (**+30%** damage), disadvantage (**-30%** damage), or no
effect, depending on their affinity with a particular biome. To summarise these combat modifiers for each troop type:
⚔️️ ️️️**Knights** excel in forests, leveraging cover and mobility, but suffer reduced effectiveness in deserts,
beaches, and snow-covered regions where their heavy armour and weapons can make it difficult to move on the soft ground
cover. Knights are equally advantaged, disadvantaged or not affected across all biome types.
🏹 **Crossbowmen** benefit from open seas and unstable terrains (such as beaches and snow), where they can remain static
and make the most of their ranged capabilities. However, they will be disadvantaged in flat, open terrain with no cover
or opportunity for concealment. Crossbowmen are the least advantaged, but also the least disadvantaged by biome
modifiers of the three troop types.
🐴 ️**Paladins** dominate open, flat terrain where mounted units thrive, but are hindered in dense forests and on open
water where their mounted capabilities are negated. They are the most advantaged, but also the most disadvantaged troop
type. In combination with the biome effects on stamina use, Paladins are less versatile than other Knights or
Crossbowmen, but are devastatingly effective in the right situations.
**Note: The scorched biome applies +30% damage to all troop types, representing the additional casualties sustained from
battling in an inhospitable environment.**
---
file: docs/pages/eternum/military/troop-tiers.mdx
meta: {}
import { TroopTiers, TroopTierDamageStats } from "@/components/TroopTiers";
import { MaxStaminaTable } from "@/components/TroopMovementTable";
# 🛡️ Troop Tiers
Troops in Eternum come in three distinct tiers: T1, T2, and T3. Tier 1 (T1) represents basic troops, while T2 and T3
troops are more advanced, offering significantly increased combat strength and stamina.
Higher-tier troops are produced by constructing specialized buildings that require rare resources and lower-tier troops
as inputs:
* Two T1 troops combine to produce one T2 troop
* Two T2 troops combine to produce one T3 troop
Each troop tier provides escalating advantages in terms of damage output and stamina.
---
file: docs/pages/eternum/realm-and-villages/buildings.mdx
meta: {}
import BuildingCard from "../../../components/BuildingCard";
import { formatNumberWithCommas } from "@/utils/formatting";
import { CapacityConfig } from "@bibliothecadao/types";
import { ETERNUM_CONFIG } from "@/utils/config";
# 🪓 Buildings
## Standard vs. Simple
Season 1 offers two building systems. Lords can switch between standard and simple building modes at any time by
clicking the toggle at the top of the construction tab.
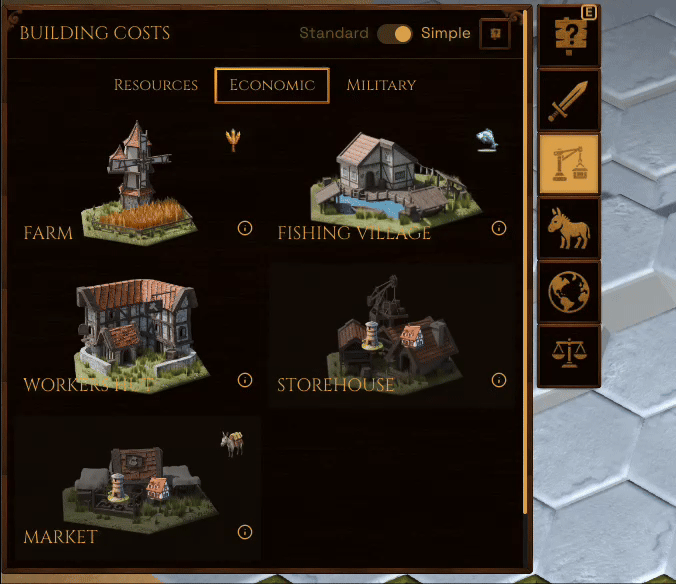
Note: Some buildings only require food to construct. Farms, Fishing Villages and Resource Buildings have the same
construction cost in both standard and simple building modes.
### Standard Mode
This is the classic Eternum experience. Construction requires a combination of labor and a particular set of resources
as building materials. Standard mode is more complex as it involves a greater number of materials in storage, however it
is far more efficient overall. This building mode is generally designed for those with multiple Realms and/or Villages
working with economies of scale.
### Simple Mode
This mode allows players to utilize Labor, a new material for Season 1, as the singular input for construction. Simple
mode greatly improves accessibility and simplifies gameplay overall, but is much less efficient in both time and
materials. This would be the preferred system for players with smaller holdings and limited access to a variety of
resources.
## Placement & Population
### Placement
The construction tab can be accessed in the Local view. Buildings can be constructed on any buildable hex of a Realm or
Village, provided the owner has the requisite materials and population capacity. Buildable hexes are defined by the
upgrade level (i.e. Settlement, City, Kingdom, or Empire) and are visible as hexes with patches of bare, prepared earth.
Most buildings can be constructed in either simple or standard mode, which toggled at the top of the construction tab.
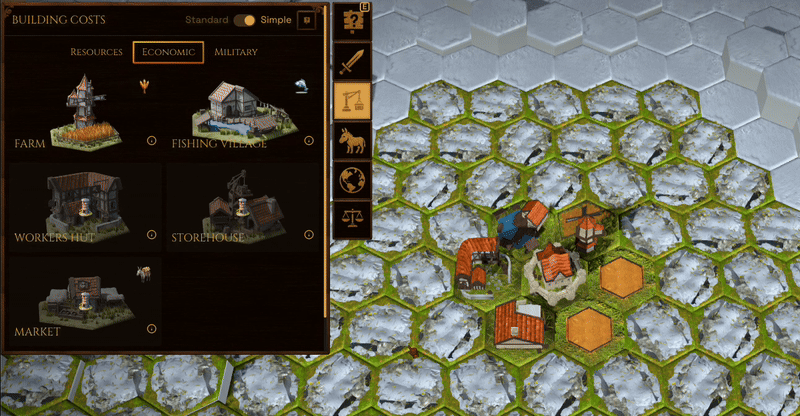
### Population
Population is the total population utilised by the currently constructed production buildings on a Realm or Village,
while the population capacity is the maximum available population with which to construct production buildings. Worker
Huts can be built to increase population capacity, while all other buildings require population capacity to be available
in order to construct. Lords must balance their construction to ensure there is enough population capacity available to
construct their desired production buildings.
## Building Types
### The Keep
Settling any Realm or Village will automatically construct a structure in the centre of your buildable hexes, known as
the Keep. This structure represents your seat of power and the central point of governance, and facilitates key
functions such as Realm/Village upgrades and Labor production. This building cannot be destroyed and provides a baseline
population capacity of 5.
### Resource Buildings
There is a specific resource building for each of the 22 resources in the game, so Lords will require separate resource
buildings if they wish to produce more than one type of resource. These buildings only require food to construct and
have the same requirements in simple and standard mode.
### Economic Buildings
These buildings define the utility of a Realm or Village, including food production, donkey production, population
capacity, and storage capacity. All of these buildings can be constructed in either standard or simple mode, but have
varying costs and population requirements:
\[economic building table, including population cost / population capacity increase (Worker Hut) / storage capacity
increase (Storehouse)]
### Military Buildings
Your military buildings are essential for training up armies to defend your territories and project your might. There
are three 'tiers' of each of the three troop types, but a different building is required to produce each troop tier. As
such, there are a total of 9 military buildings in the game.
Note: Only T1 troop buildings can be constructed in both standard and simple mode. T2 and T3 military buildings are only
available for construction in standard mode.
### Same Building Cost Increase
Building multiple buildings of the same type will cost progressively more each time. The cost increase is calculated
using the following formula:
```
Cost = Base Cost + (Base Cost × 0.5 × (N-1)²)
```
Where:
* **Base Cost** is the initial cost of the building (from the tables above)
* **N** is the number of the same buildings already constructed
For example, if you build a second building of the same type (N=2), the cost will be:
```
Cost = Base Cost + (Base Cost × 0.5 × (2-1)²)
= Base Cost + (Base Cost × 0.5 × 1)
= Base Cost × 1.5
```
Note: Buildings need to be exactly the same type for this rule to apply. For example, building a Stone Quarry after a
Lumber Camp (both being resource buildings) would not be subject to this cost increase.
---
file: docs/pages/eternum/realm-and-villages/realm.mdx
meta: {}
import { StartingResources, StructureType } from "@/components/StartingResources";
import { StartingMilitary } from "@/components/StartingMilitary";
import { RealmDefenseSlotsTable } from "@/components/DefendingArmies";
import { BuildableHexes } from "@/components/BuildableHexes";
import { RealmLeveling } from "@/components/RealmLeveling";
import { importantNote } from "@/components/styles";
# 🏰 Realms
The 8,000 Realms are the foundation of Eternum, each uniquely destined to shape the fate of this vast continent. As
limited and irreplaceable strongholds, these Realms serve as the primary playing pieces of the game. Each Realm settled
in Eternum can produce between one and seven different resources, depending on the metadata of the original Realm NFT
and its associated Season Pass. This metadata also aligns each Realm to one of sixteen ancient Orders, however the
meaning of this alignment has been lost to the mist… for now.
### Settling
To settle a Realm, Lords must first burn a Season Pass, which gives them the ability to select the location of their
homeland from an array of locations scattered across the map. For Season 1, there is a seven-day settling period before
the game starts, allowing players to organise and position themselves before a move can be made. Realms can still be
settled after this period, however the earlier in the season that Lords can establish their holdings, the greater their
production potential and overall experience.
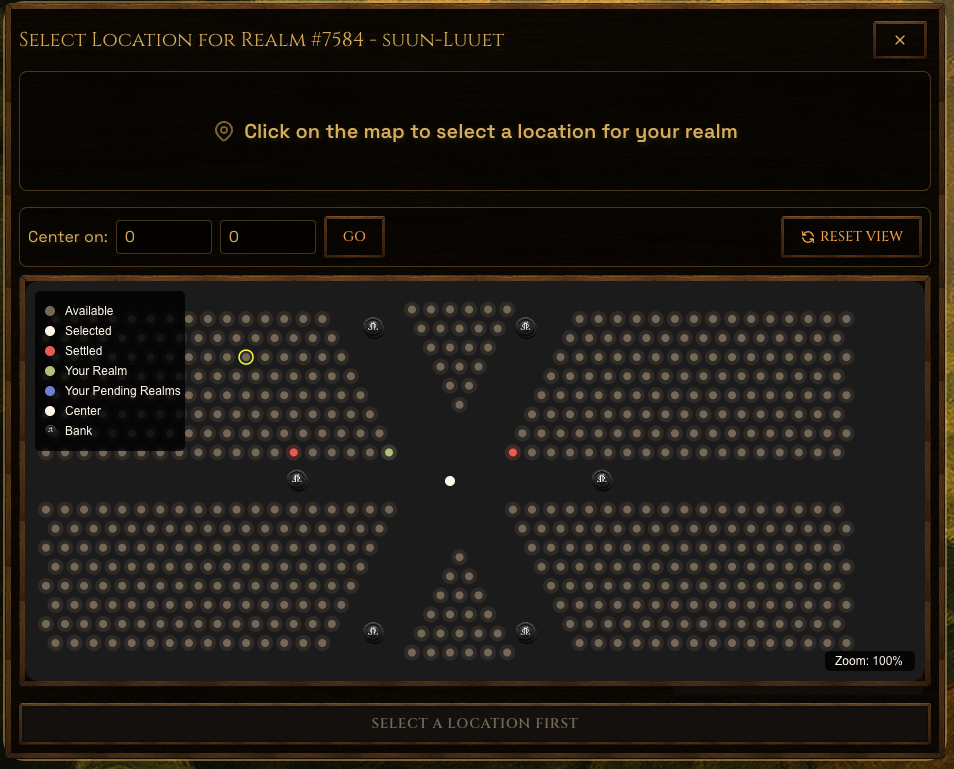
⚠️ IMPORTANT NOTE
When the game starts, all structures are immune to attacks and raids for 24 hours. Once the first day of gameplay
has concluded, there are no more instances of immunity, all Realms and Villages settling into the game after this
time will need to rely on their starting defences and consider their starting locations carefully. Lords entering
the game after the immunity period are advised to make use of the spectator mode to scout for relatively safe
spawning locations before initiating a settling transaction.
### Starting Resources
### Starting Military
Each Realm starts with the majority of the starting troops deployed to the first defense slot as a guard army. A small
number of troops remain within the Realm's storage to be deployed as a field army should the Realm Lord wish to explore
beyond their walls. Troops can be transferred from the guard army to the field army using the troop transfer process
explained in the [Movement & Exploration](/eternum/worldmap-movement/movement) section. The specific type of military
unit is determined by your Realm's biome, and is strategically chosen to have a combat advantage in that particular
environment.
### Realm Progression
Realms can grow from a Settlement into a City, then to a Kingdom, and finally an Empire. Each upgrade level unlocks
additional buildable hexes and defensive army slots, allowing for the expansion of your economy and strengthening of
your defences. Each progression requires specific materials, outlined in the table below:
---
file: docs/pages/eternum/realm-and-villages/villages.mdx
meta: {}
import ResourceProbability from "@/components/ResourceProbability";
import { StartingResources, StructureType } from "@/components/StartingResources";
import { StartingMilitary } from "@/components/StartingMilitary";
# 🛖 Villages
Beyond the limited number of sacred Realms lies the humble yet vital Village—small settlements established on hexes that
surround a settled Realm. These modest outposts offer a more accessible entry into Eternum, serving as gateways for new
settlers and loyal subjects. Additionally, they can act as supplementary playing pieces for more serious players who
wish to maximise their potential. Each settled Realm can have up to six Villages surrounding it, allowing up to 48,000
Villages in Season 1!
### Settling
Villages are settled by minting a Village token. Aspiring settlers can choose to place their Village next to a specific
Realm by entering the asset ID of a settled Realm, or let fate guide them to a random Realm's outskirts - either way,
the Realm that they spawn adjacent to becomes that Village's 'parent' Realm. Players may also choose which of the six
possible hexes they spawn on by selecting a compass direction.
*Three villages settled next to a realm*
### Starting Resources
### Starting Military
Each Village starts with the majority of the starting troops deployed to the first defense slot as a guard army. A small
number of troops remain within the Village’s storage to be deployed as a field army should the Village Lord wish to
explore beyond their walls. Troops can be transferred from the guard army to the field army using the troop transfer
process explained in the [Movement & Exploration](/eternum/worldmap-movement/movement) section. The specific type of
military unit is determined by your Village's biome, and is strategically chosen to have a combat advantage in that
particular environment.
### Resource Roll
When minting a Village, a random number is rolled to determine the single resource that it can produce.
### Limitations
Villages produce food, resources, troops, donkeys, and labor at 50% the rate of Realms. They can receive and purchase
food, labor, donkeys and resources without restriction, but can only receive troops from their parent Realm - this
limitation is in place to prevent players from spawning a Village on their rival's Realm and 'teleporting' troops there
by using the donkey network.
### Village Progression
Villages have a limited progression path and may only upgrade once from Settlement to City. The cost and benefits of
this progression is the same as that of a Realm.
---
file: docs/pages/eternum/realm-and-villages/wonders.mdx
meta: {
"title": "Wonders",
"description": "The Wonders of Realms Eternum"
}
# ✨ Wonders
Of the 8,000 Realms, 50 are distinguished by the presence of Wonders—unique and magical places of power that confer
special benefits, making them highly desirable playing pieces that may put a target on their owner's back.
In Season 1, Wonder Realms grant a 20% production bonus not only to themselves but also to all Realms and Villages
located within 12 tiles. This bonus means the Wonder Realm itself, the surrounding Realms (up to six), and all Villages
settled around these seven Realms produce food, resources, troops, labor, and donkeys at 120% efficiency compared to
standard Realms, using the same inputs.
Key Notes:
* Production rates are not increased by this bonus, more is produced overall for the same amount of input.
* These bonuses do not stack. There is no benefit to settling multiple wonders in a limited range.
---
file: docs/pages/eternum/resources/automation.mdx
meta: {}
# Resource Automation
Resource automation allows players to set up continuous production processes and automated transfers within their realms
and between entities. This system helps manage resource generation and distribution efficiently without constant manual
intervention.
## Overview
The automation system works by processing two types of "orders" that you define:
1. **Production Orders**: Automate resource production within your realms
2. **Transfer Orders**: Automate resource transfers between your entities (realms, villages, hyperstructures, etc.)
Both types of orders specify what you want to automate, the method or conditions, target amounts or schedules, and
priorities.
**Key Features:**
* **Order-Based**: Define specific production and transfer tasks.
* **Priority System**: Control the sequence of execution for multiple orders.
* **Multiple Automation Types**: Choose between production automation and transfer automation.
* **Entity-Specific**: Automation is configured on a per-entity basis.
* **Browser Dependent**: Automation processes run in your browser; the game tab must remain open for automation to
function.
* **10-Minute Cycles**: All automation is processed every 10 minutes.
## How It Works
The automation system periodically checks and processes active orders for each entity.
1. **Processing Interval**: All orders are evaluated every 10 minutes.
2. **Order Prioritization**: For each entity, orders are sorted by their priority number (1 being the highest).
3. **Pause Functionality**: You can pause all automation for any entity, which will skip all orders for that entity.
4. **Order Processing**: The system processes different order types:
### Production Order Processing
* **Balance Check**: Before executing, the system checks if the realm has sufficient input resources or labor.
* **Recipe-Based Production**: Production follows predefined recipes from the game's configuration.
* **Cycle Calculation**: Determines the maximum number of production cycles possible based on available inputs.
* **Transaction**: If conditions are met, a blockchain transaction is initiated to perform the production.
### Transfer Order Processing
* **Schedule Check**: For recurring transfers, checks if enough time has passed since the last transfer.
* **Threshold Check**: For conditional transfers, checks if resource levels meet the specified conditions.
* **Resource Availability**: Verifies the source entity has sufficient resources for the transfer.
* **Transaction**: If conditions are met, initiates a transfer transaction between entities.
## Production Types
There are three types of production automation:
1. **Standard (Resource-to-Resource)**:
* Converts one or more input resources into a different output resource.
* Example: Using Wood and Stone to produce Tools.
2. **Simple (Labor-to-Resource)**:
* Utilizes Labor from the realm to produce a specific resource.
* Example: Using Labor to harvest Food.
3. **Resource-to-Labor**:
* Converts a specified resource into Labor for the realm.
* Example: Burning Food to generate Labor.
## Production Order Modes
Production orders can operate in two different modes:
1. **Produce Once**:
* Produces resources until the target amount is reached, then stops.
* Shows progress as "Produced / Target" (e.g., "1,500 / 5,000").
* Can be set to "Infinite" to produce continuously without a limit.
2. **Maintain Balance**:
* Keeps resource balance at the target level.
* Production triggers when balance drops below target minus buffer percentage.
* Example: Target 10,000 with 10% buffer = production starts when balance drops below 9,000.
* Useful for maintaining steady resource levels.
## Transfer Types
Transfer automation allows you to automatically move resources between your entities. There are three transfer modes:
1. **Recurring Transfers**:
* Transfers resources at regular intervals.
* Minimum interval: 10 minutes (due to automation cycle).
* Example: Transfer 1,000 Food from Realm A to Village B every 2 hours.
* *\[GIF placeholder: Setting up a recurring transfer]*
2. **Maintain Stock Transfers**:
* Transfers when the destination entity falls below a threshold.
* Example: Transfer 500 Wood to Village when it has less than 1,000 Wood.
* Helps keep important entities well-supplied.
* *\[GIF placeholder: Setting up a maintain stock transfer]*
3. **Depletion Transfers**:
* Transfers when the source entity exceeds a threshold.
* Example: Transfer excess Stone when Realm has more than 5,000 Stone.
* Prevents resource overflow and distributes surplus.
* *\[GIF placeholder: Setting up a depletion transfer]*
## Managing Automation Orders (UI)
You can manage automation orders through the "Automation" tab within any entity's interface, or through the global "All
Automations" view.
### Production Automation
Production automation is managed within individual realm interfaces.
*\[GIF placeholder: Navigating to realm automation tab]*
#### Adding a New Production Order
1. Click the "Add New Automation" button.
2. Configure the following:
* **Order Mode**:
* `Produce Once`: Produce up to target amount then stop
* `Maintain Balance`: Keep resource balance at target level
* **Production Type**:
* `Standard (Resource-based)`
* `Simple (Labor-based)`
* `Resource to Labor`
* **Priority (1-9)**:
* Set the execution priority. `1` is the highest priority, `9` is the lowest.
* **Resource to Produce / Resource Input for Labor**:
* Select the resource you want to produce or consume.
* The UI shows required input resources for Resource-to-Resource recipes.
* **Target Amount**:
* For "Produce Once": Set total quantity to produce, or check "Infinite" for continuous production.
* For "Maintain Balance": Set the balance level to maintain.
* **Buffer Percentage** (Maintain Balance only):
* Production starts when balance drops below target minus buffer percentage.
3. Click "Add Automation".
*\[GIF placeholder: Creating a production automation order]*
### Transfer Automation
Transfer automation allows you to move resources between any of your entities.
*\[GIF placeholder: Accessing transfer automation]*
#### Adding a New Transfer Order
1. **Select Source Entity**:
* Choose entity type (Your Realms, Your Villages, etc.)
* Search and select the specific source entity
2. **Configure Transfer**:
* **Transfer Mode**:
* `Recurring`: Transfer at regular intervals
* `Maintain Stock`: Transfer when destination is low
* `Depletion Transfer`: Transfer when source is high
* **Destination Entity**:
* Choose entity type and select specific destination
* **Resources to Transfer**:
* Add multiple resources with specific amounts
* **Schedule/Conditions**:
* For Recurring: Set interval in minutes (minimum 10 minutes)
* For conditional transfers: Set threshold amounts
3. Click "Add Transfer Automation".
*\[GIF placeholder: Creating a transfer automation order]*
#### Transfer Interval Guidelines
* **Minimum Interval**: 10 minutes (matches automation processing cycle)
* **Common Intervals**:
* 10m, 30m, 1h, 2h, 6h, 24h (preset buttons available)
* Custom intervals can be set in minutes
* **Effective Timing**: Transfers occur when automation cycles align with your interval
### Viewing and Managing Orders
#### Individual Entity View
Each entity shows its automation orders in a table with:
* **Priority**: Order execution priority
* **Mode**: "Once", "Maintain", or transfer mode ("Recurring", "Stock", "Depletion")
* **Resource**: Visual representation of resource flow
* **Target/Balance**: Target amounts or transfer schedules
* **Produced**: Amount produced (production orders only)
* **Type**: Order type (production or transfer)
* **Actions**: Remove button
*\[GIF placeholder: Viewing automation orders in entity interface]*
#### Global Automation View
The "All Automations" view shows orders from all entities:
* **Realm Filter**: Filter by specific realm or view all
* **Order Status**: Visual indicators for completed, paused, or active orders
* **Countdown Timer**: Shows time until next automation cycle
* **Bulk Actions**: Pause/resume or delete orders
*\[GIF placeholder: Using the global automation view]*
### Pausing Automation
You can pause automation at different levels:
1. **Individual Entity**: Pause all automation for a specific entity
2. **Individual Order**: Remove specific orders
3. **Global Control**: Manage all orders from the global view
*\[GIF placeholder: Pausing and resuming automation]*
## Important Considerations
### General Requirements
* **Browser Must Be Open**: Automation processing occurs in your browser. If you close the game tab or your browser,
automation will stop.
* **10-Minute Processing Cycle**: All automation is processed every 10 minutes. This affects timing for all orders.
* **Transaction Costs**: Each successful automation cycle that results in production or transfer will involve a
blockchain transaction, which incurs network transaction fees.
* **Processing Time**: Due to the 10-minute processing interval and potential network conditions, there might be a delay
before an order is executed or its results are visible.
### Production Automation Considerations
* **Resource Availability**: Production orders will only execute if the required input resources are available in the
realm at the time of processing.
* **Recipe Dependencies**: Production follows predefined game recipes. You cannot produce resources that don't have
valid recipes.
* **Storage Limits**: Produced resources will increase your realm's balance and may cause resource loss if storage is
full.
### Transfer Automation Considerations
* **Entity Ownership**: You can only set up transfers between entities you own.
* **Transfer Timing**:
* Minimum effective interval is 10 minutes due to processing cycle
* Intervals shorter than 10 minutes will effectively transfer every 10 minutes
* Non-10-minute multiples may have irregular timing based on when transfers align with processing cycles
* **Resource Availability**: Transfers will only execute if the source entity has sufficient resources at processing
time.
* **Multiple Resources**: You can transfer multiple different resources in a single transfer order.
* **Conditional Logic**: Maintain Stock and Depletion transfers check conditions each cycle and only transfer when
thresholds are met.
### Best Practices
1. **Start Simple**: Begin with basic production orders or simple recurring transfers to understand the system.
2. **Monitor Initially**: Watch your first few automation cycles to ensure orders work as expected.
3. **Use Priorities**: Set appropriate priorities when you have multiple orders to control execution sequence.
4. **Plan for Delays**: Account for the 10-minute processing cycle when planning time-sensitive operations.
5. **Check Resource Flows**: Regularly verify that your automated transfers are maintaining desired resource
distributions.
*\[GIF placeholder: Monitoring automation performance and adjusting orders]*
---
file: docs/pages/eternum/resources/bridging.mdx
meta: {}
import { EfficiencyTable } from "@/components/EfficiencyTable";
# 🌀 Bridging (Portals)
Portals provide Lords with the ability to bridge materials into and out of Eternum, connecting the in-game economy with
the Starknet infrastructure layer. Portals are mysterious and unstable devices, so they tend to burn up most of the
materials sent through. However, there is evidence that the power of the Hyperstructures can increase the stability of
Portals throughout Eternum. As Lords manage to reestablish these mighty structures throughout the land, the efficiency
of bridging in and out of Eternum improves for all.
Each Realm comes equipped with a Portal. Realm Lords are able to bridge materials both into and out of their Realms
directly. Villages, however, have more limited bridging capabilities—they can bridge materials out of Eternum, but not
in. This is in line with their existing restrictions on receiving troops from external sources. They are also limited to
utilizing the Portal belonging to their parent Realm, for which the Realm owner collects a 5% fee on all materials and
tokens bridged out of the game.
---
file: docs/pages/eternum/resources/production.mdx
meta: {}
import {
StandardTroopProduction,
SimpleTroopProduction,
SimpleResourceProduction,
StandardResourceProduction,
LaborProduction,
DonkeyProduction,
} from "@/components/ResourceProduction";
# ⚒️ Production
## Standard vs. Simple
As is the case for building construction, Lords can choose between standard and simple production systems when deciding
how they wish to produce their materials. This can be done by selecting the appropriate panel when making an order in
the production screen.
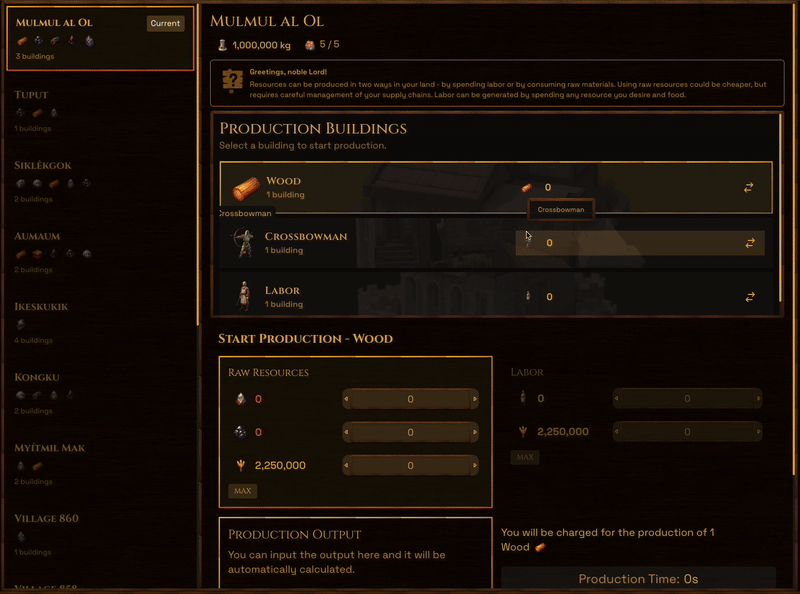
*Note: Production works a little differently in Season 1 compared to Season 0. Instead of streaming resources unit by
unit and producing an output for as long as you have the requisite inputs, Lords must now commit the full material
requirements to the production order upfront. The output is then produced at a given rate per second.*
### Standard Mode
The classic Eternum system—every resource and troop production order requires food and a combination of other resources
as inputs. As with buildings, standard mode is the more complex but efficient way to produce materials.
### Simple Mode
Simple mode reduces the requirements for a production order to food and labor. This is, once again, less efficient in
both time and resources—but it allows Lords to produce resources on their Realm or Village even if they don't have
access to the input resources required in standard mode.
## Materials Production
### Food
The rich and fertile lands of Eternum produce two staple food sources, Wheat and Fish. These are the only materials that
can be produced without inputs, so once a Farm or Fishing Village has been constructed, they can produce food
indefinitely.
### Resources
The 22 resources found in Eternum are the backbone of the world economy. Producing one resource requires the input of
two other resources (standard mode), or labor (simple mode). Resources are also the main components in building
construction, troop production and Hyperstructure contributions - so mastery of these assets is key to success and
security.
### Labor
Labor is a new mechanic in Season 1 that has been introduced to simplify and supplement gameplay. It is the primary
input for buildings and production in simple mode, but is also required in standard mode for building construction,
Realm upgrades and Hyperstructure contribution.
Labor is produced by permanently burning resources in the central structure of a Realm or Village. This creates a steady
stream of Labor at a fixed rate. Each of the 22 resources can be burned to produce Labor at varying efficiency,
dependent on rarity. The amount of resources burned per second to produce Labor at the fixed rate is as follows:
### Troops
Troops are essential for exploration, defending your lands and conquering your enemies. Every Lord will be required to
procure troops, either through production or purchase, to ensure they remain a relevant force throughout the game. In
Season 1, two new troop tiers have been introduced, each requiring a combination of food, rare resources, and troops of
the previous tier as input for production.
Note: Only T1 troops can be produced in both standard and simple mode. T2 and T3 troops can only be produced in standard
mode.
### Donkeys
The humble steeds that move all materials around Eternum must themselves be produced in a market building. Donkeys are
one-time-use and consumed after a single journey, so Lords will need to procure a steady supply of donkeys, either
through production or purchase, to ensure that they can sustain their supply chains, trade with other players, and buy
and sell from the Banks. Since donkeys are the 'gas' of this onchain world, they consume $LORDS - the native token of
the ecosystem - during production.
---
file: docs/pages/eternum/resources/resources.mdx
meta: {}
import RarityResourceTable from "@/components/RarityResourceTable";
import ResourceIcon from "@/components/ResourceIcon";
import TroopsLimitTable from "@/components/TroopsLimitTable";
import { ResourcesIds } from "@bibliothecadao/types";
# 🧱 Materials
## Materials Overview
Materials encompass all transportable assets in Eternum, including:
* **Resources**: The 22 distinct resource types that can be produced on Realms based on their metadata
* **Food**: Essential wheat and fish that fuel production and troop movement
* **Troops**: Military units (Knights, Crossbowmen, Paladins) that can be assigned to armies
* **Donkeys**: One-time-use transport units required for moving materials across the world
* **Labor**: A special material that enables "simple mode" construction and production
* **Ancient Fragments**: Rare materials found in-game that are critical for Hyperstructure construction
While all materials can be transported, only specific resources can be produced by Realms based on their inherent
properties. Most materials (except Labor) can be bridged out as ERC20 tokens.
## Resources
Resources are the foundation of **Eternum**'s economy. Each of the 22 resources plays a vital role in the game's
ecosystem, from basic production to advanced military operations. All in-game resources can be traded freely and bridged
out as ERC20 tokens.
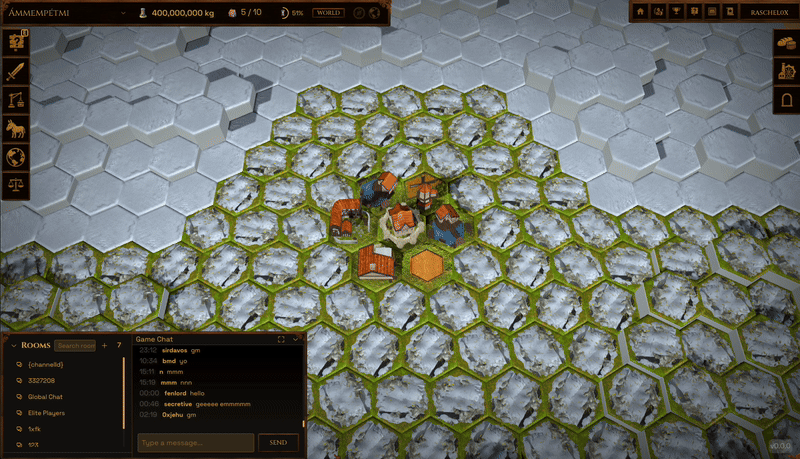
## Material Categories
#### Material Tiers
Resources are distributed across the 8,000 Realms based on rarity, creating natural scarcity and trade opportunities.
### Basic Materials
#### Food
Food is the cornerstone of your realm's economy:
* Produced without additional resource inputs
* Required for most production chains
* Essential for maintaining troops and construction
### Strategic Materials
#### Military
Military units have unique properties:
* They can be traded as resources
* Cannot be converted back to tradeable form once deployed to an army
* Once deployed, they can still be transferred between armies if they are located on adjacent hexes and are of the same
type and tier
#### Transport (Donkeys)
Essential for logistics:
* Required for transferring and trading materials
* Consumed after a single use
### Special Materials
#### **$LORDS** Currency
**$LORDS** is Eternum's economic backbone:
* Used as the currency for all market transactions
* Bridgeable to external networks
* Required to produce donkeys
#### Labor
Labor is a fundamental production material:
* New Season 1 mechanic
* Can be produced by any Realm or Village
* Introduces ‘simple mode’ which reduces the complexity of resource and troop production
* Required to construct most buildings
* Produced by burning resources, with rarer resources yielding more labor
#### Ancient Fragments
Strategic end-game material:
* Only found in world map Fragment Mines
* Required to commence the construction of Hyperstructures
* Cannot be produced
---
file: docs/pages/eternum/resources/storage.mdx
meta: {}
import { WeightTable } from "@/components/WeightTable";
import { formatNumberWithCommas } from "@/utils/formatting";
import { divideByPrecision } from "@/utils/resources";
import { CapacityConfig, ResourcesIds } from "@bibliothecadao/types";
import { ETERNUM_CONFIG } from "@/utils/config";
# 🏺 Storage System
Your available storage limits the amount of materials your Realm or Village can hold. Effective management of your
storage capacity is essential for successful development. Each Realm and Village begins with a base capacity of
**{formatNumberWithCommas(ETERNUM_CONFIG().carryCapacityGram[CapacityConfig.Storehouse]/1000)} kg**. You'll need to
construct storehouses to expand your storage capabilities.
## Material Weights
Materials in Eternum have different weights that affect storage:
## Storehouses
Expand your storage capacity to support your growing realm:
* **Capacity**: Each storehouse adds
{formatNumberWithCommas(ETERNUM_CONFIG().carryCapacityGram[CapacityConfig.Storehouse]/1000 )}kg of storage capacity.
* **Expansion**: Build additional storehouses to increase total storage.
* **Danger**: When storage is full new production is wasted
## World Structure Storage
All world structures have set storage capacities **(that cannot be expanded through the use of storehouses)**. These
capacities are as follows:
| Structure Type | Storage Capacity |
| -------------- | ------------------------------------------------------------------------------------------------------------ |
| Hyperstructure | {formatNumberWithCommas(ETERNUM_CONFIG().carryCapacityGram[CapacityConfig.HyperstructureStructure]/1000)} kg |
| Bank | {formatNumberWithCommas(ETERNUM_CONFIG().carryCapacityGram[CapacityConfig.BankStructure]/1000)} kg |
| Fragment Mine | {formatNumberWithCommas(ETERNUM_CONFIG().carryCapacityGram[CapacityConfig.FragmentMineStructure]/1000)} kg |
## Storage Management Tips
> 💡 **Best Practices**
>
> * Check storage levels regularly
> * Build new storehouses before reaching capacity
> * Pause production when storage is nearly full
> * Plan material usage to maximize storage efficiency
---
file: docs/pages/eternum/resources/transfers-and-trade.mdx
meta: {}
import { importantNote } from "@/components/styles";
# 📜 Transfers & Trade
## Transport
### Transfers
Throughout the season, Lords will face the need to transfer materials from place to place around the world map, whether
it be to their own Realms and Villages, or other structures like Banks, Hyperstructures, and Fragment Mines. Lords have
two primary options for initiating transfers:
* **Detailed Transfer**: Players can use the comprehensive transfer menu accessible from the Trade tab. This allows
transfers of multiple types of materials simultaneously, to any structure on the map—including those belonging to
other players.
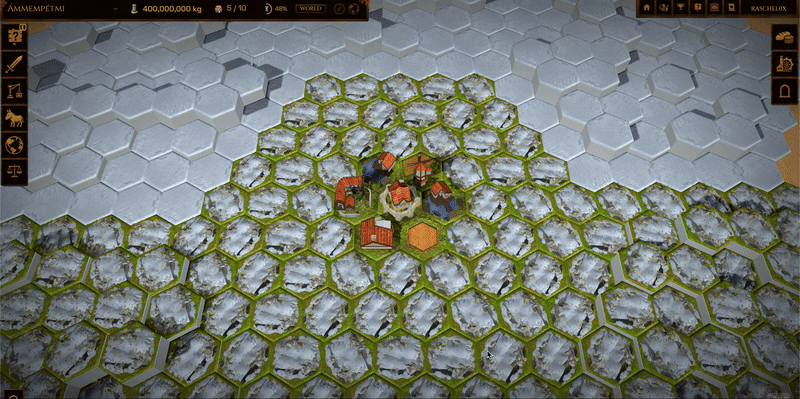
* **Quick Transfer**: Alternatively, Lords can swiftly transfer resources between their own structures using the
resources sidebar, ideal for managing internal logistics and maintaining supply chains.
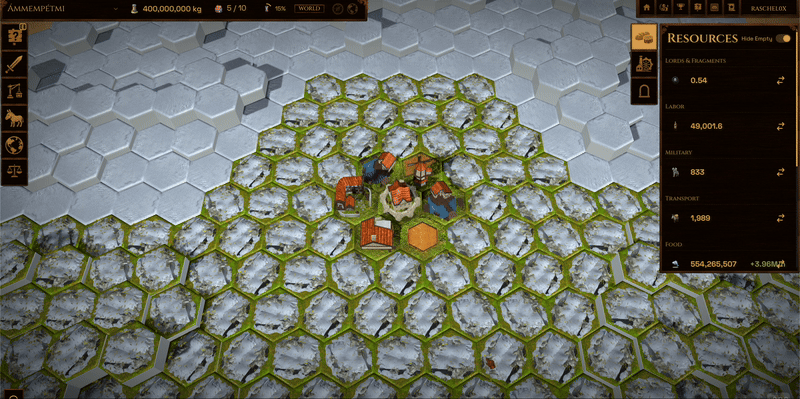
### The Donkey Network
When a transfer is initiated, donkeys travel through the invisible and immutable donkey network. This is the key
infrastructure upon which all material transport takes place, facilitating trade between players and Banks. One donkey
is capable of carrying up to 500 kg of materials, so the number of donkeys required for a transfer depends on the total
weight of the materials being moved. Once a transfer is initiated, it cannot be cancelled, and the journey will take
some time depending on the distance to the destination structure. Upon arrival, transferred materials and donkeys must
be manually claimed via the 'Resource Arrivals' tab before they become available for use.
⚠️ IMPORTANT NOTE
In Season 1, resource arrivals are restricted by 'gates' to assist in reducing the load that resource arrival
entities have on the game. As donkeys arrive at their destination bearing their various goods, they need to wait
until the start of a new Eternum Day for the structure's arrival gate to open before they can enter and be claimed
by the owning Lord. For example, if a transfer arrives at 2:56 p.m. the owner of the structure will only need to
wait until 3:00 p.m. for the transfer to become claimable, however if the transfer arrives at 3:02 p.m., the goods
won't be claimable until the next Eternum Day starts (4 p.m.).
## Banks & Marketplaces
### Banks
Six ancient Banks stand evenly distributed around, and equidistant from, the centre of the map.
At the start of the game, each Bank is occupied by powerful groups of bandits. Lords who conquer a Bank become the
beneficiary of any fees from the Automated Market Maker (AMM) trades that they facilitate. Although Banks collect fees
independently, they collectively share liquidity, ensuring consistent and stable trading conditions throughout Eternum.
Realms and Villages automatically utilize their nearest Bank when trading through the AMM.
### AMM (Automated Market Maker)
The Automated Market Maker (AMM) is a decentralized trading protocol facilitated by the six Banks, allowing for
instantaneous and trustless trades 24/7—the DeFi of Eternum. Trades executed via the AMM automatically utilize the
closest Bank, directing trade fees specifically to that Bank's owner. Lords can further benefit from the AMM by
contributing materials and $LORDS to liquidity pools, earning a share of the fees generated from subsequent swaps. Lords
should be aware of the relatively high fees for using the AMM and consider the Orderbook for other trading options
first.
### Orderbook
Complementing the AMM, the Orderbook is a peer-to-peer marketplace, allowing Lords to place precise buy or sell orders
at chosen quantities and prices. This traditional method of trading incurs lower fees and offers Lords strategic
control, enabling them to manage market conditions to their advantage and set OTC orders for friends and allies.
---
file: docs/pages/eternum/worldmap-movement/movement.mdx
meta: {}
import TroopMovementTable from "@/components/TroopMovementTable";
import { BiomeStamina } from "@/components/BiomeStamina";
# 🧭 Movement & Exploration
## Stamina
Troops in Eternum are deployed onto the world map as armies. Army movement and actions across the map are governed by
stamina, a critical resource Lords must carefully manage.
Stamina is expended whenever an army moves between hexes, explores previously unrevealed areas, or launches an attack
against enemy forces. Each of these actions has a specific stamina cost, making strategic planning essential to
maximizing an army's effectiveness. Armies regenerate stamina at a consistent rate of +20 stamina per Eternum Day. Each
troop type possesses different maximum stamina capacities, outlined in the table below:
Lords should remain cautious about fully depleting the stamina of their armies, as forces with low stamina may find
themselves severely disadvantaged if attacked by enemy forces.
## Movement
Moving armies in the client
Armies in Eternum can move freely into any adjacent, unoccupied hex. A hex is considered occupied if it contains a
Realm, Village, another army, or a world structure such as a Bank, Fragment Mine, or Hyperstructure. Armies may also
move to more distant hexes, provided there is a continuous path of adjacent, unoccupied hexes leading to the
destination, and the army has sufficient stamina available for the entire journey.
The amount of stamina consumed when moving into a hex depends on both the biome of that hex and the type of troops
within the army. The table below details biome-specific stamina costs for each troop type:
## Exploring
When an army is positioned adjacent to an unexplored hex, Lords may choose to send that army forth to uncover what lies
hidden. Exploring an unknown hex always costs 30 stamina and will reveal that hex to all Lords, permanently expanding
the known boundaries of the world.
If the newly explored hex contains a world structure or an agent army, the exploring army will automatically be pushed
back to the hex from which they started without any additional stamina penalty. Additionally, during exploration, armies
gather any valuables they encounter, receiving a stack of randomly selected resources directly into their inventory. If
an army becomes overloaded, they will not be able to undertake further exploration, but can continue to travel on
previously-explored hexes. Armies can return to a structure to offload the materials, or drop them to free up space.
Note that any materials discarded in the field will be permanently lost (burned).
Given the possibility of encountering hostile agent armies during exploration, Lords should ensure that their exploring
armies are well-prepared and capable of defending themselves in unexpected combat situations.
Explore in the client
## Troop Transfers
Lords may transfer troops from one army to another, provided that they are on adjacent hexes, the same troop type, and
the same tier. To transfer troops from one army to another, or to a structure, the player first needs to select its army
on the worldmap using left-click, then right-click on the target and select the Transfer -> Transfer Troops tab.
Transferring troops from one army to another with lower stamina makes no change to the stamina of either army.
Transferring from an army with lower stamina to an army with higher stamina will reduce the higher-stamina army to equal
that of the lower stamina army.
Transfer troops in the client
---
file: docs/pages/eternum/worldmap-movement/worldmap.mdx
meta: {}
# 🗺️ The World Map
## An Unexplored World
At the beginning of each season, Eternum lies entirely unexplored, shrouded in mystery and opportunity. The only
exceptions are the six ancient Banks, whose location and surrounding hexes are known and visible from the outset. They
can be seen when settling and when observing the world view, each equidistant from the centre of the map and from one
another.
When Lords settle a Realm, the six hexes directly adjacent to the Realm hex and the six possible village locations are
automatically revealed if they haven't already been explored. Beyond these instances, the world remains cloaked by the
mist, waiting for ambitious Lords to send forth their armies to explore. Once a hex is explored, the state of the hex
(i.e. the biome and whether it contains a world structure or army) is permanently revealed to all Lords.
## Biomes
Each hex is assigned one of 16 biome types, each with unique aesthetics and strategic implications. These biomes are
procedurally generated across the world map following specific rules that dictate their distribution, ensuring a
coherent yet varied world, with certain biomes naturally occurring more or less frequently than others.
Biomes impact both combat effectiveness and troop movement. Each troop type—Knights, Crossbowmen, and Paladins—can
potentially be advantaged or disadvantaged in battle depending on the biome in which combat takes place; this is
explained in more detail in the [Military section](/eternum/military/stamina-and-biomes). Additionally, biomes have the
potential to enhance or impede army maneuvers, as the various biomes impact the stamina consumption of each troop type
differently as they move between hexes—this is explained further in the
[Movement & Exploration](/eternum/worldmap-movement/movement) section below.